What is the Noise Protocol
Introduction to the Noise Protocol Framework
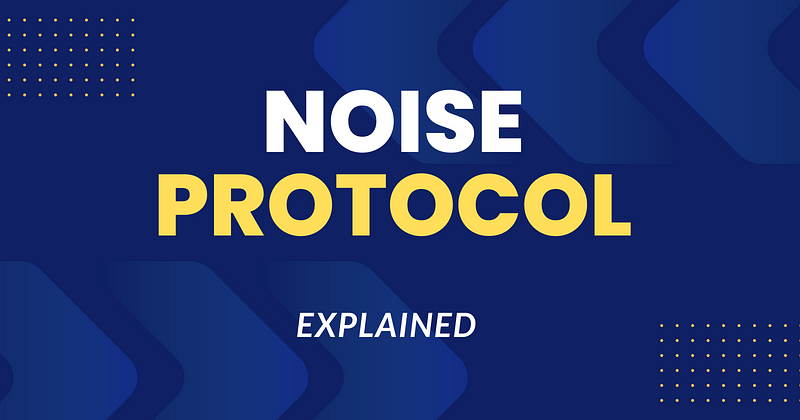
Introduction to the Noise Protocol Framework
Background
The Noise Protocol Framework was developed by Trevor Perrin, a notable name in cryptography, primarily known for his work on the Signal Protocol. It was designed to simplify the creation of secure communication protocols by offering modular components.
Core Concepts
The Noise Protocol is not a single protocol but a framework. It provides building blocks for constructing custom protocols tailored to specific needs. These building blocks include cryptographic primitives like Diffie-Hellman key exchange, symmetric encryption, and hash functions.
Components of the Noise Protocol
1. Handshake Patterns
Noise defines several handshake patterns. These patterns dictate how two parties establish a secure connection. Key patterns include:
- XX: A mutual authentication pattern.
- IK: An optimized pattern for scenarios where one party already possesses the other’s static public key.
- Noise Pipes: A variant used by WireGuard VPN for enhanced privacy.
2. Cryptographic Primitives
Noise uses a set of well-established cryptographic primitives:
- Diffie-Hellman (DH): For key exchange.
- Symmetric Encryption: AESGCM or ChaChaPoly for encrypting messages.
- Hash Functions: Like SHA256 or BLAKE2, for generating hash values during the handshake and encrypting messages.
3. Protocol Negotiation
Noise allows for dynamic protocol negotiation, letting parties agree on a particular set of cryptographic primitives and handshake patterns.
Handshake Patterns in Detail
Handshake patterns are central to the Noise Protocol. They define how two parties, typically referred to as the initiator and the responder, exchange cryptographic keys and establish a secure connection.
Key Patterns:
- XX Pattern: This is a mutual authentication pattern where both parties exchange and authenticate their public keys during the handshake. It’s ideal for scenarios where prior identity knowledge isn’t assumed.
- IK Pattern: This pattern is used when the initiator already knows the responder’s static public key, allowing for fewer message exchanges and a faster handshake.
- Noise Pipes: An adaptation of the IK pattern, Noise Pipes are used in WireGuard to establish secure VPN connections with fewer round-trips.
Cryptographic Primitives
The Noise Protocol uses a set of cryptographic primitives to ensure the confidentiality, integrity, and authenticity of messages.
- Diffie-Hellman (DH) Key Exchange:
- Essential for establishing a shared secret between parties without prior interaction.
- Noise supports several DH functions like Curve25519 and Curve448.
2. Symmetric Encryption:
- For message confidentiality and integrity.
- Noise typically uses AES-GCM or ChaCha20-Poly1305.
3. Hash Functions:
- Used for generating hash values during handshakes and deriving keys.
- Common choices include SHA256 and BLAKE2.
Protocol Negotiation
Protocol negotiation in Noise allows parties to agree on a set of cryptographic primitives and handshake patterns dynamically. This feature is crucial for flexibility and future-proofing the protocol against advances in cryptography.
- Modularity: Different components (like DH, cipher functions, and hash functions) can be mixed and matched.
- Forward Secrecy: Noise supports forward secrecy, a feature ensuring that the compromise of long-term keys does not compromise past session keys.
Hands-on Examples with Rust
Implementing the Noise Protocol in Rust can be a rewarding experience, given Rust’s focus on safety and performance. The Rust ecosystem has libraries like snow
, which is a Rust implementation of the Noise Protocol Framework. Below are some basic examples of how to use snow
to implement different aspects of the Noise Protocol. Keep in mind that these examples are simplified and intended for educational purposes.
Setting Up snow
in Rust
First, you need to include snow
in your Cargo.toml
:
[dependencies]
snow = "0.7.1"
Basic Noise Protocol Implementation
1. Creating a Noise Builder
This example shows how to create a Noise protocol builder with the XX handshake pattern and default cryptographic choices.
use snow::params::NoiseParams;
use snow::Builder;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let params: NoiseParams = "Noise_XX_25519_ChaChaPoly_BLAKE2s".parse()?;
let builder = Builder::new(params);
// Continue with building the initiator and responder...
Ok(())
}
2. Initiator and Responder Handshake
This demonstrates a basic handshake between an initiator and a responder.
fn main() -> Result<(), Box<dyn std::error::Error>> {
let params: NoiseParams = "Noise_XX_25519_ChaChaPoly_BLAKE2s".parse()?;
let mut initiator = Builder::new(params.clone()).build_initiator()?;
let mut responder = Builder::new(params).build_responder()?;
// Initiator creates first message
let mut msg_buf = [0u8; 512];
let len = initiator.write_message(&[], &mut msg_buf)?;
// Responder reads the message and responds
let mut response_buf = [0u8; 512];
let _ = responder.read_message(&msg_buf[..len], &mut response_buf)?;
let len = responder.write_message(&[], &mut msg_buf)?;
// Initiator finalizes the handshake
let _ = initiator.read_message(&msg_buf[..len], &mut response_buf)?;
// Handshake is complete, proceed to secure communication
Ok(())
}
3. Secure Message Transmission
After the handshake, both parties can securely exchange encrypted messages.
fn secure_message_exchange() -> Result<(), snow::Error> {
let mut initiator = /* ... */;
let mut responder = /* ... */;
// Assuming handshake is completed...
// Initiator encrypts a message
let mut msg = b"Hello, Responder!".to_vec();
let mut encrypted_msg = vec![0u8; msg.len() + 16]; // extra space for tag
initiator.write_message(&msg, &mut encrypted_msg)?;
// Responder decrypts the message
let mut decrypted_msg = vec![0u8; encrypted_msg.len()];
responder.read_message(&encrypted_msg, &mut decrypted_msg)?;
println!("Decrypted message: {:?}", String::from_utf8_lossy(&decrypted_msg));
Ok(())
}
Error Handling and Security Considerations
- Ensure proper error handling in real-world implementations.
- Always verify the integrity and authenticity of messages in secure communication.
- Be aware of the security properties of the chosen handshake pattern and cryptographic primitives.
Security Features
- Identity Hiding: Certain handshake patterns in Noise can hide participants’ identities until their authenticity is verified.
- Resistance to Replay Attacks: Noise protocols are designed to be resistant to replay attacks, where an attacker retransmits a valid data transmission to replicate a function or action.
Operational Mechanics of Noise Protocol
Handshake State Machine
- Initializing State: The handshake begins with initializing states, where each party sets up its cryptographic keys (both static and ephemeral).
- Interactive Handshaking: Parties exchange messages according to the chosen handshake pattern. This may involve multiple rounds of communication.
- Transition to Transport Phase: Once the handshake is completed, the protocol transitions to the transport phase, where encrypted messages can be exchanged securely.
Key Compromise Implications
- Session Keys vs. Static Keys: Noise differentiates between ephemeral session keys and long-term static keys. Compromise of a session key does not affect past sessions (forward secrecy) but may affect future sessions if static keys are compromised.
- Rotating Keys: To enhance security, Noise protocols often implement key rotation mechanisms, ensuring long-term security even in prolonged communication sessions.
Advanced Cryptographic Concepts in Noise
Zero-Knowledge Proofs
Noise can incorporate zero-knowledge proofs to authenticate without revealing any additional information, enhancing privacy and security.
Post-Quantum Cryptography
While not inherently a part of Noise, the framework’s modularity allows for the integration of post-quantum cryptographic algorithms to safeguard against future quantum-computing threats.
Cross-Protocol Attacks
Noise’s design minimizes the risk of cross-protocol attacks by ensuring that handshake state is cleanly separated from transport state, and by using distinct nonce spaces for different handshake messages.
🚀 Explore a Wealth of Resources in Software Development and More by Luis Soares
📚 Learning Hub: Expand your knowledge in various tech domains, including Rust, Software Development, Cloud Computing, Cyber Security, Blockchain, and Linux, through my extensive resource collection:
- Hands-On Tutorials with GitHub Repos: Gain practical skills across different technologies with step-by-step tutorials, complemented by dedicated GitHub repositories. Access Tutorials
- In-Depth Guides & Articles: Deep dive into core concepts of Rust, Software Development, Cloud Computing, and more, with detailed guides and articles filled with practical examples. Read More
- E-Books Collection: Enhance your understanding of various tech fields with a series of free e-Books, including titles like “Mastering Rust Ownership” and “Application Security Guide” Download eBook
- Project Showcases: Discover a range of fully functional projects across different domains, such as an API Gateway, Blockchain Network, Cyber Security Tools, Cloud Services, and more. View Projects
- LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- Medium: Read my articles on Medium and give claps if you find them helpful. It motivates me to keep writing and sharing Rust content. Follow on Medium
- Personal Blog: Discover more on my personal blog, a hub for all my Rust-related content. Visit Blog
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- Twitter: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis.soares@linux.com
Senior Software Engineer | Cloud Engineer | SRE | Tech Lead | Rust | Golang | Java | ML AI & Statistics | Web3 & Blockchain