Using Channels in Rust: Why and When?
Hey there! If you’re diving into the world of concurrent programming with Rust, you’ve probably come across the term “channels”. But what…
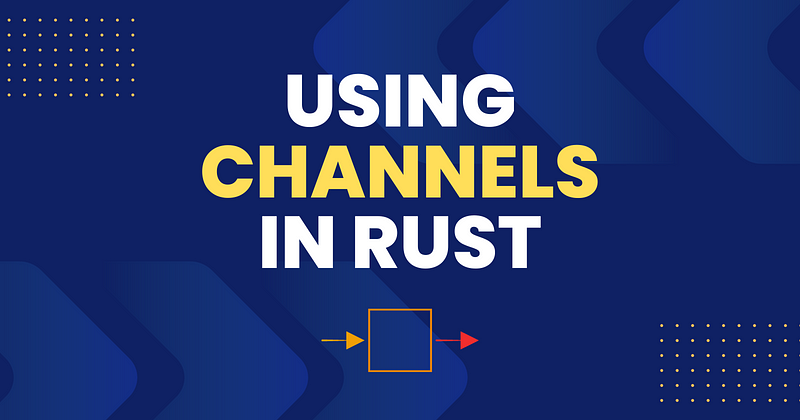
Hey there! If you’re diving into the world of concurrent programming with Rust, you’ve probably come across the term “channels”. But what are they, exactly? Why would you use them, and when? In this article, we’ll explore channels in Rust, break down their functionality, and guide you through scenarios where they shine. By the end, you’ll have a solid grasp on why and when to use channels in your Rust projects. Let’s get started!
Do not share state - exchange messages
The philosophy “Do not share state; exchange messages” is a core principle in concurrent and parallel programming, especially when we’re discussing systems with multiple threads or processes. Let’s break this down and understand its significance:
Problems with Shared State
When multiple threads or processes have access to shared mutable state:
- Data Races: These occur when two or more threads access shared data concurrently, and at least one of them modifies it. This can lead to unpredictable behavior and hard-to-diagnose bugs.
- Complex Synchronization: Guarding shared state against concurrent access typically requires the use of synchronization primitives like mutexes or semaphores. While effective, they introduce complexity and can be error-prone when not used correctly.
- Deadlocks: These arise when two or more threads are blocked forever, each waiting for the other to release a resource. This typically happens due to incorrect synchronization around shared state.
- Performance Bottlenecks: When multiple threads are contending to access a shared resource, it can lead to performance degradation, negating the benefits of concurrency.
Message Passing as a Solution
Instead of directly sharing state, threads/processes communicate by sending and receiving messages. This has several advantages:
- Decoupling: The sender and receiver are decoupled. The sender doesn’t need to know about the receiver’s internal state or logic. This makes the system modular and more maintainable.
- Avoiding Concurrency Issues: Since there’s no shared mutable state, the risks of data races and related concurrency issues are significantly reduced.
- Scalability: Message-passing systems can often be more scalable since threads or processes can operate independently and communicate asynchronously.
Think of message passing like a mail system. Instead of multiple people trying to simultaneously write on a single piece of paper (shared state), each person sends a letter (message) containing their information. The recipient can then process each letter independently and in order.
The Anatomy of Channels
- Components of a Channel: At its core, a channel consists of two main components:
- Sender (or Transmitter): This component is responsible for sending messages into the channel.
- Receiver: This component is tasked with reading messages from the channel.
Here’s a simple example:
use std::thread;
use std::sync::mpsc; // "mpsc" stands for "multiple producer, single consumer".
fn main() {
let (tx, rx) = mpsc::channel();
thread::spawn(move || {
tx.send("Hello from the spawned thread!").unwrap();
});
let received = rx.recv().unwrap();
println!("Main thread received: {}", received);
}
In this code, the spawned thread sends a message, and the main thread waits for and receives it.

Types of Channels
Channels in Rust primarily come in two flavors, which are differentiated by their behavior with regards to their capacity: Unbounded Channels and Bounded Channels.
Unbounded Channels
Unbounded channels do not have any capacity limit. This means you can keep sending messages to an unbounded channel without it ever blocking due to being full. However, this also means that there’s a risk of using an unbounded amount of memory if the receiver can’t keep up with the sender.
Code Example:
use std::thread;
use std::sync::mpsc;
fn main() {
let (tx, rx) = mpsc::channel(); // This creates an unbounded channel by default
thread::spawn(move || {
for i in 1..10 {
tx.send(i).unwrap();
thread::sleep(std::time::Duration::from_millis(100));
}
});
for received in rx {
println!("Received: {}", received);
}
}
Bounded Channels
Bounded channels have a fixed capacity. When the channel is full, any attempt to send a new message will block until there’s space. Bounded channels can be used to implement backpressure, ensuring that a fast producer does not overwhelm a slower consumer.
Code Example:
use std::thread;
use std::sync::mpsc;
fn main() {
let (tx, rx) = mpsc::sync_channel(2); // Bounded channel with a capacity of 2
thread::spawn(move || {
for i in 1..10 {
tx.send(i).unwrap();
println!("Sent: {}", i);
thread::sleep(std::time::Duration::from_millis(100));
}
});
thread::sleep(std::time::Duration::from_millis(500)); // Sleep for demonstration
for received in rx {
println!("Received: {}", received);
thread::sleep(std::time::Duration::from_millis(500));
}
}
In this example, you’ll notice that after sending two messages, the sender will block for half a second before it can send another, due to the receiver processing messages at a slower rate.
Channel Behavior and Characteristics
Blocking and Non-blocking Operations:
- A call to
send
can be non-blocking, which means it pushes the message into the channel and immediately returns. However, if using a bounded channel,send
can block if the channel is full. - On the other hand, the
recv
call is blocking by default. It waits for a message to be available. If you don't want to block, you can usetry_recv
, which attempts to retrieve a message and returns immediately, regardless of whether a message is available.
Single Consumer: The “mpsc” in std::sync::mpsc
stands for "multiple producers, single consumer". This means you can have multiple senders transmitting messages into the channel but only one receiver taking messages out. This design decision simplifies the internal synchronization requirements of the channel.
Closing Channels: When the sender is dropped, it indicates that no more messages will be sent on the channel. The receiver can then determine when it has received all messages by checking for the Err
variant from the recv
method.
Error Handling: If a sender tries to send data after the receiver has been dropped, it will result in an error. Similarly, if a receiver tries to receive data after all senders have been dropped and the channel is empty, it will also result in an error.
let’s create an example that simulates a simple scenario: Multiple worker threads will generate random numbers and send them to a main thread, which will collect and display them.
use std::thread;
use std::sync::mpsc;
use std::time::Duration;
use rand::Rng; // For generating random numbers. You'll need to add the `rand` crate to your dependencies.
const NUM_WORKERS: usize = 3;
const NUM_MESSAGES_PER_WORKER: usize = 5;
fn main() {
// Create a channel. tx for transmitting and rx for receiving.
let (tx, rx) = mpsc::channel();
// Start multiple worker threads.
for id in 0..NUM_WORKERS {
let thread_tx = tx.clone();
thread::spawn(move || {
let mut rng = rand::thread_rng();
for _ in 0..NUM_MESSAGES_PER_WORKER {
let num: i32 = rng.gen_range(0..100); // Generate a random number between 0 and 99.
println!("Worker {} sending number: {}", id, num);
// Send the generated number to the main thread.
thread_tx.send(num).unwrap();
// Sleep for a short duration.
thread::sleep(Duration::from_millis(50));
}
});
}
// In the main thread, receive and print numbers from worker threads.
for _ in 0..(NUM_WORKERS * NUM_MESSAGES_PER_WORKER) {
let received = rx.recv().unwrap();
println!("Main thread received: {}", received);
}
}
In this example, we’re using the rand
crate to generate random numbers, so you'll need to add it to your Cargo.toml
if you're trying this example out:
[dependencies]
rand = "0.8"
You can adjust the constants NUM_WORKERS
and NUM_MESSAGES_PER_WORKER
to control the number of worker threads and how many messages each sends, respectively.
This program will spawn NUM_WORKERS
threads, and each will generate and send NUM_MESSAGES_PER_WORKER
random numbers to the main thread, which then displays them.
When and Why to Use Channels
1. Producer-Consumer Pattern
The Producer-Consumer pattern is a classic concurrency model where one or more threads (producers) generate data and place it in a buffer or queue, and one or more other threads (consumers) process or consume the data from that buffer or queue.
Why use channels in this pattern?
- Data Safety: Channels ensure safe data transfer without data races or inconsistencies. The ownership system in Rust ensures data transferred via channels can’t be accessed simultaneously by multiple threads.
- Synchronization: Channels inherently provide synchronization. For instance, if a consumer tries to fetch data from an empty channel, it’ll block until data is available.
2. Task Parallelism
Task parallelism involves breaking a larger task into smaller sub-tasks that can be processed concurrently, usually to accelerate the completion of the entire task.
Why use channels here?
- Aggregating Results: After processing sub-tasks concurrently, results often need to be aggregated. Channels allow worker threads to send their results back to the main thread or a collecting thread.
- Dynamic Task Distribution: If tasks aren’t uniformly distributed, worker threads can use channels to request more tasks from a central dispatcher or return tasks they can’t handle.
3. Event-driven Architectures
In event-driven architectures, various parts of a system respond to events generated elsewhere. This pattern is common in GUI applications, servers, and many other contexts.
Why channels?
- Decoupling: Channels decouple the event producer from the consumer. An event source can send events without knowing or caring about the details of the event handlers.
- Ordering: Channels maintain the order of events, ensuring they are handled in the order they are sent.
4. Signal Handling
Threads often need a way to be notified of external conditions or signals. For example, a thread might need to be interrupted, informed of certain system-level events, or asked to reload its configuration.
Why channels for signal handling?
- Clean Termination: Instead of abruptly terminating threads (which can lead to resource leaks or inconsistent states), channels can be used to send a termination signal, allowing threads to wrap up and terminate gracefully.
- Configuration Updates: Channels can carry configuration payloads. If a system’s configuration changes, the new configuration can be sent via a channel to the concerned thread, which can then adjust its behavior accordingly.

Wrapping Up
In essence, channels in Rust (and similar constructs in other languages) are more than just data transfer mechanisms. They play an integral role in designing systems where different parts need to communicate concurrently, efficiently, and safely. The abstractions provided by channels help reduce the inherent complexities and pitfalls of multi-threaded programming.
Check out some interesting hands-on Rust articles!
🌟 Developing a Fully Functional API Gateway in Rust — Discover how to set up a robust and scalable gateway that stands as the frontline for your microservices.
🌟 Implementing a Network Traffic Analyzer — Ever wondered about the data packets zooming through your network? Unravel their mysteries with this deep dive into network analysis.
🌟 Building an Application Container in Rust — Join us in creating a lightweight, performant, and secure container from scratch! Docker’s got nothing on this.
🌟 Implementing a P2P Database in Rust: Today, we’re going to roll up our sleeves and get our hands dirty building a Peer-to-Peer (P2P) key-value database.
🌟 Building a Function-as-a-Service (FaaS) in Rust: If you’ve been exploring cloud computing, you’ve likely come across FaaS platforms like AWS Lambda or Google Cloud Functions. In this article, we’ll be creating our own simple FaaS platform using Rust.
🌟 Building an Event Broker in Rust: We’ll explore essential concepts such as topics, event production, consumption, and even real-time event subscriptions.
Read more articles about Rust in my Rust Programming Library!
Visit my Blog for more articles, news, and software engineering stuff!
Follow me on Medium, LinkedIn, and Twitter.
Leave a comment, and drop me a message!
All the best,
Luis Soares
CTO | Tech Lead | Senior Software Engineer | Cloud Solutions Architect | Rust 🦀 | Golang | Java | ML AI & Statistics | Web3 & Blockchain