Understanding and Preventing Short Address Attacks in Solidity Smart Contracts
What is a Short Address Attack?
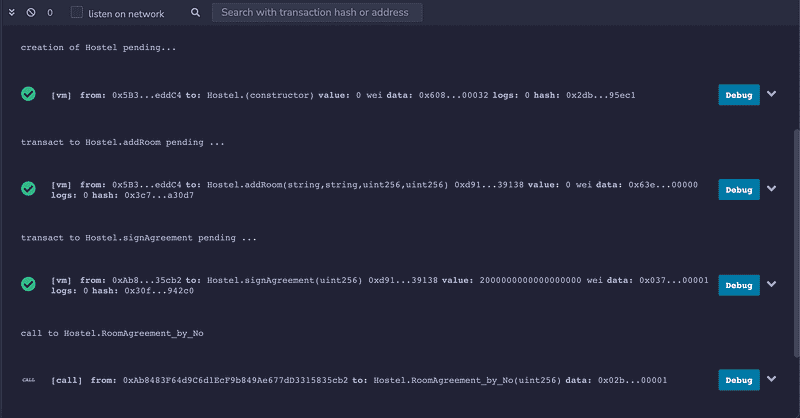
What is a Short Address Attack?
A Short Address Attack occurs when an attacker manipulates the data sent in an Ethereum transaction, tricking the smart contract into reading more data than was sent. This exploit leverages the way Solidity pads shorter arguments to 32 bytes.
Ethereum addresses are typically 20 bytes long, but if an attacker uses an address shorter than 20 bytes, the Solidity contract will automatically pad the difference on the right side with zeros to ensure that the address is appropriate. Suppose a function expects two arguments (e.g., an address and a token amount). In that case, the contract might interpret part of the second argument (the token amount) as part of the first (the address) due to the automatic padding, effectively allowing the attacker to send more tokens than intended.
An Example of a Short Address Attack
Consider a Solidity function transfer(address _to, uint256 _value)
that is used to transfer tokens from the sender's account to another account. Here's a simplified version:
function transfer(address _to, uint256 _value) public {
require(balances[msg.sender] >= _value);
balances[msg.sender] -= _value;
balances[_to] += _value;
emit Transfer(msg.sender, _to, _value);
}
This function works correctly in normal circumstances. However, an attacker could send a transaction from an Ethereum address only 19 bytes long instead of 20 bytes. If the transaction was sent in raw form, it might look like this:
0xa9059cbb000000000000000000000000f17f52151ebef6c7334fad080c5704d77216b7320000000000000000000000000000000000000000000000000000000000000064
The first part (0xa9059cbb
) is the function selector. The next part is the 19-byte address, followed by the 32-byte representation of the number of tokens to send (100 in this case).
The contract would read the 20-byte address by combining the 19-byte address with the first byte of the 32-byte number of tokens. This effectively causes the transfer of 256 times more tokens than intended because the first byte of the 32-byte gets multiplied by 256.
Preventing Short Address Attacks
Several strategies can help prevent Short Address Attacks:
- Input validation: One of the most straightforward and effective solutions is to validate the length of the address within the contract. This can be done by adding a
require()
statement to check that the address is 20 bytes long:
function transfer(address _to, uint256 _value) public {
require(_to.length == 20);
require(balances[msg.sender] >= _value);
balances[msg.sender] -= _value;
balances[_to] += _value;
emit Transfer(msg.sender, _to, _value);
}
2. Safe math libraries: Safe math libraries like OpenZeppelin's SafeMath
can also help prevent these types of attacks. They provide functions that perform mathematical operations with safety checks that throw errors when overflows or underflows occur. For example:
using SafeMath for uint256;
function transfer(address _to, uint256 _value) public {
require(_to.length == 20);
require(balances[msg.sender] >= _value);
balances[msg.sender] = balances[msg.sender].sub(_value);
balances[_to] = balances[_to].add(_value);
emit Transfer(msg.sender, _to, _value);
}
3. Secure programming practices: Ensure all functions' arguments are of a known length. Avoid using the msg.data.length
in your code, as it may leave room for manipulation.
4. Off-chain verification: Wallets and other user-facing applications can also add checks to ensure users send transactions to full-length addresses.
5. Auditing and testing: Regularly auditing smart contracts and rigorous testing can help identify such security vulnerabilities. The use of automated auditing tools and manual code reviews can prevent such attacks.
Short Address Attacks represent a fascinating case study of the type of nuanced vulnerabilities in the blockchain world. They underscore the importance of robust, secure coding practices and rigorous testing to ensure the integrity of smart contracts. By utilizing input validation, secure libraries, and adhering to secure programming practices, developers can mitigate the risks of these attacks, safeguarding both their applications and their users' assets.
Stay tuned, and happy coding!
Visit my Blog for more articles, news, and software engineering stuff!
Follow me on Medium, LinkedIn, and Twitter.
Check out my most recent book — Application Security: A Quick Reference to the Building Blocks of Secure Software.
All the best,
Luis Soares
CTO | Head of Engineering | Blockchain Engineer | Web3 | Cyber Security | Solidity | Smart Contracts
#blockchain #solidity #security #attacks #defense #ethereum #ethereum #smartcontracts #network #datastructures #data #smartcontracts #web3 #security #privacy #confidentiality #cryptography #softwareengineering #softwaredevelopment #coding #software