Six Rust Crates for Networking
Today, we’re exploring six popular Rust crates in Rust network programming. From handling low-level socket operations with Socket2 to…
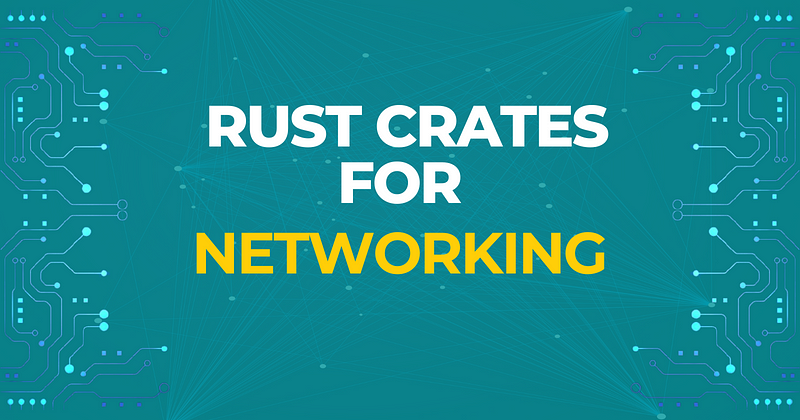
Today, we’re exploring six popular Rust crates in Rust network programming. From handling low-level socket operations with Socket2 to routing URLs at lightning speed with Matchit, each of these crates brings something unique to the table.
Whether you’re building a microservice with Tonic-build or setting up secure HTTP connections with Hyper-rustls, these tools have got you covered. Let’s unwrap these crates and see what makes them stand out!
1. Socket2 Crate
Socket2 stands out as a versatile crate for network socket manipulation. It’s designed for those who need more control over network interactions than what standard libraries offer.
Why Opt for Socket2?
- Direct Socket Manipulation: Offers detailed control over socket behavior.
- Cross-Platform Functionality: Ensures compatibility across various operating systems.
- Comprehensive Configuration Options: Supports extensive customization for networking operations.
Key Features
- Support for Multiple Domains: Includes IPv4 and IPv6.
- Diverse Socket Types: Handles TCP, UDP, and other socket types.
- Non-Blocking Mode: Facilitates asynchronous operations with non-blocking sockets.
- Detailed Socket Control: Allows setting options like timeouts, TTL, and more.
Explained Code Examples
Creating a TCP Socket
use socket2::{Socket, Domain, Type, Protocol};
// Create a new TCP socket in the IPv4 domain
let socket = Socket::new(Domain::IPV4, Type::STREAM, Some(Protocol::TCP))?;
// This line creates a TCP socket for IPv4 communication.
Configuring Socket Options
use std::time::Duration;
// Set a read timeout of 10 seconds
socket.set_read_timeout(Some(Duration::from_secs(10)))?;
// Set a write timeout of 10 seconds
socket.set_write_timeout(Some(Duration::from_secs(10)))?;
// These lines configure the socket to time out if a read or write operation takes longer than 10 seconds.
Binding and Listening to a Port
use std::net::SocketAddr;
// Define the address and port to bind to
let address: SocketAddr = "127.0.0.1:8080".parse()?;
socket.bind(&address.into())?;
socket.listen(128)?;
// This code binds the socket to the local address 127.0.0.1 on port 8080 and sets it to listen for incoming connections, with a backlog of 128 connections.
To dive deeper into Socket2 and unlock its full potential, explore its documentation and resources on Crates.io.
2. Ipnet Crate
The Ipnet crate in Rust is designed for managing and manipulating IP network addresses, simplifying tasks related to IP networking.
Why Choose Ipnet?
- IP Address Handling: Streamlines operations involving IPv4 and IPv6 addresses.
- Subnet Management: Facilitates working with network subnets, a common requirement in network programming.
- CIDR Operations: Supports operations with Classless Inter-Domain Routing (CIDR), crucial for modern network design and management.
Key Features
- IpNet Types: Offers specialized types like
IpNet
,Ipv4Net
, andIpv6Net
. - Prefix and Subnet Operations: Allows for efficient handling of network prefixes and subnets.
- Range Calculations: Provides functionality for calculating the range of IP addresses in a network.
Explained Code Examples
Creating an IPv4 Network
use ipnet::Ipv4Net;
// Parse a CIDR string into an Ipv4Net type
let network = "192.168.1.0/24".parse::<Ipv4Net>()?;
// This line creates an IPv4 network representing the subnet 192.168.1.0/24.
Checking for Network Membership
use std::net::Ipv4Addr;
// Check if an IP address belongs to the defined network
let addr = Ipv4Addr::new(192, 168, 1, 10);
let is_member = network.contains(&addr);
// This code checks whether the IP address 192.168.1.10 is part of the previously defined network.
Iterating Over a Network
// Iterate over all IP addresses in the network
for ip in network.hosts() {
println!("{}", ip);
}
// This loop iterates through and prints all host addresses in the 192.168.1.0/24 network.
For more detailed information and advanced features, check out Ipnet’s documentation on Crates.io.
3. Tonic-build Crate
Tonic-build is a Rust crate specifically designed to enhance the experience of working with gRPC (Google’s Remote Procedure Call) in Rust applications. It’s a part of the Tonic ecosystem, which is known for its high-performance gRPC framework.
Why Use Tonic-build?
- Automatic Code Generation: Simplifies the process of generating Rust code from gRPC definitions.
- Integration with Tonic: Designed to seamlessly work with Tonic, providing a smooth workflow for Rust-based gRPC services.
- Protocol Buffers Support: Fully supports Protocol Buffers (protobuf), the default interface definition language for gRPC.
Key Features
- Streamlined Workflow: Automates the boilerplate code generation process.
- Custom Configuration: Offers options to customize the code generation according to specific needs.
- Compatibility: Works well with other parts of the Tonic ecosystem and supports asynchronous programming in Rust.
Explained Code Examples
Defining a gRPC Service (Proto File)
First, you need to define your gRPC service in a Protocol Buffers (.proto) file. Here’s a simple example:
syntax = "proto3";
package myapp;
service MyService {
rpc SayHello (HelloRequest) returns (HelloReply);
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
Generating Rust Code
To generate Rust code from this definition, use Tonic-build in your build.rs
file:
fn main() -> Result<(), Box<dyn std::error::Error>> {
tonic_build::compile_protos("proto/myapp.proto")?;
Ok(())
}
// This will generate Rust code for the defined service and messages when building the project.
For deeper insights and advanced usage, you can explore the Tonic-build documentation on Crates.io.
4. Tower-http Crate
Tower-http is a Rust crate that provides middleware, utilities, and additional components for HTTP clients and servers. It is part of the Tower ecosystem, known for its service-oriented framework.
Why Use Tower-http?
- Middleware Solutions: Offers a range of middleware components for various HTTP-related tasks.
- Asynchronous Support: Fully supports asynchronous operations, a key feature in modern Rust development.
- Modularity: Its modular design allows for easy integration and customization within different HTTP applications.
Key Features
- Comprehensive Middleware Library: Includes features like request/response logging, compression, authorization, and more.
- Easy Integration: Designed to work seamlessly with popular Rust HTTP frameworks.
- Performance Enhancements: Optimizes HTTP server and client performance through various middleware.
Explained Code Examples
Adding Logging Middleware
use tower_http::trace::TraceLayer;
use tower::ServiceBuilder;
// Create a service stack with the TraceLayer for logging
let service = ServiceBuilder::new()
.layer(TraceLayer::new_for_http())
.service(my_http_service);
// This adds HTTP request and response logging to the service.
Using Compression Middleware
use tower_http::compression::CompressionLayer;
// Add compression middleware to the service stack
let service = ServiceBuilder::new()
.layer(CompressionLayer::new())
.service(my_http_service);
// This enables response compression for supported formats like gzip or brotli.
For more detailed information, visit Tower-http’s documentation on Crates.io.
👏 Your Support Matters: If you enjoy the content, please give it claps on Medium and share the content away! Your support encourages me to continue creating and sharing valuable Rust resources.
5. Hyper-rustls Crate
Hyper-rustls is a Rust crate that integrates Rustls, a modern TLS implementation, with Hyper, a popular HTTP library in Rust. This integration allows Rust developers to create HTTP clients and servers with robust, secure TLS support.
Why Use Hyper-rustls?
- Security: Rustls offers a modern, security-focused approach to TLS, avoiding legacy protocols and ciphers.
- Performance: Both Rustls and Hyper are known for their performance, making Hyper-rustls a great choice for high-speed, secure HTTP applications.
- Ease of Use: Simplifies the process of adding TLS to HTTP clients and servers in Rust.
Key Features
- Rustls Integration: Seamlessly integrates the Rustls TLS stack with Hyper’s HTTP capabilities.
- TLS Support for HTTP: Enables secure HTTPS connections for both client and server implementations.
- Modern Cryptography: Leverages Rustls’s focus on modern, secure cryptography practices.
Explained Code Examples
Setting Up a TLS-Enabled HTTP Client
use hyper::Client;
use hyper_rustls::HttpsConnector;
// Create a TLS-enabled HTTPS connector
let https = HttpsConnector::with_native_roots();
let client = Client::builder().build::<_, hyper::Body>(https);
// This code sets up an HTTPS client using Hyper-rustls, leveraging Rustls for TLS.
Creating a Secure HTTP Server
use hyper::server::Server;
use hyper_rustls::TlsAcceptor;
use tokio_rustls::TlsAcceptor;
// Assume 'config' is your Rustls server configuration
let tls_acceptor = TlsAcceptor::from(Arc::new(config));
let server = Server::bind(&addr).serve(make_svc);
// Here, a secure HTTP server is set up using Hyper with Rustls for TLS.
For more information and advanced features, check out Hyper-rustls’s documentation on Crates.io.
6. Matchit Crate
Matchit is a Rust crate designed for high-performance URL routing, making it a valuable tool for web servers and web applications in Rust.
Why Use Matchit?
- Performance: Offers fast and efficient URL matching, crucial for high-traffic web applications.
- Simple API: Provides a straightforward and easy-to-use API for URL routing.
- Zero-Copy: Implements zero-copy strategies, enhancing performance and resource efficiency.
Key Features
- Pattern Matching: Supports dynamic URL patterns with placeholders.
- Flexible Routing: Allows for versatile route definitions and matches.
- Lightweight: Focuses on routing with minimal overhead.
Explained Code Examples
Creating a Router
use matchit::Router;
let mut router = Router::new();
// Initialize a new router for URL matching.
Adding Routes
router.insert("/user/:name", "user_profile")?;
// Adds a route for user profiles, where ":name" is a dynamic segment.
Matching URLs
let matched = router.at("/user/john_doe").unwrap();
assert_eq!(matched.params["name"], "john_doe");
// Matches a URL to the defined route, extracting the dynamic "name" parameter.
For more details and to explore Matchit’s capabilities, visit its documentation on Crates.io.
Conclusion
And that’s a wrap! We’ve journeyed through six incredible Rust crates, each offering its unique flavor to the networking realm. Socket2 gave us the low-level control we crave, while Ipnet made IP address manipulation a breeze. Tonic-build streamlined our gRPC adventures, Tower-http enhanced our HTTP interactions, and Hyper-rustls ensured our connections are secure. Finally, Matchit showed us the fast lane of URL routing. As you embark on your networking projects, remember these tools and how they can elevate your Rust experience. Happy coding, and may your packets always find their way! 🚀🌐🦀
🚀 Explore More Rust Resources by Luis Soares
📚 Learning Hub: Dive into the world of Rust programming with my comprehensive collection of resources:
- Hands-On Tutorials with GitHub Repos: Get practical experience by following step-by-step tutorials, each accompanied by a dedicated GitHub repository. Access Tutorials
- In-Depth Guides & Articles: Understand key Rust concepts through detailed guides and articles, loaded with practical examples. Read More
- E-Book: “Mastering Rust Ownership”: Enhance your Rust skills with my free e-Book, a definitive guide to understanding ownership in Rust. Download eBook
- Project Showcases: Explore 10 fully functional Rust projects, including an API Gateway, Peer-to-Peer Database, FaaS Platform, Application Container, Event Broker, VPN Server, Network Traffic Analyzer, and more. View Projects
- LinkedIn Newsletter: Stay updated with the latest in Rust programming by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- Medium: Read my articles on Medium and give claps if you find them helpful. It motivates me to keep writing and sharing Rust content. Follow on Medium
- Personal Blog: Discover more on my personal blog, a hub for all my Rust-related content. Visit Blog
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- Twitter: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment, and drop me a message!
All the best,
Luis Soares
CTO | Tech Lead | Senior Software Engineer | Cloud Solutions Architect | Rust 🦀 | Golang | Java | ML AI & Statistics | Web3 & Blockchain