Rust Structs and Enums Under the Hood
Understanding a bit about the inner mechanics and how structs and enums behave regarding memory allocation and performance provides deep…
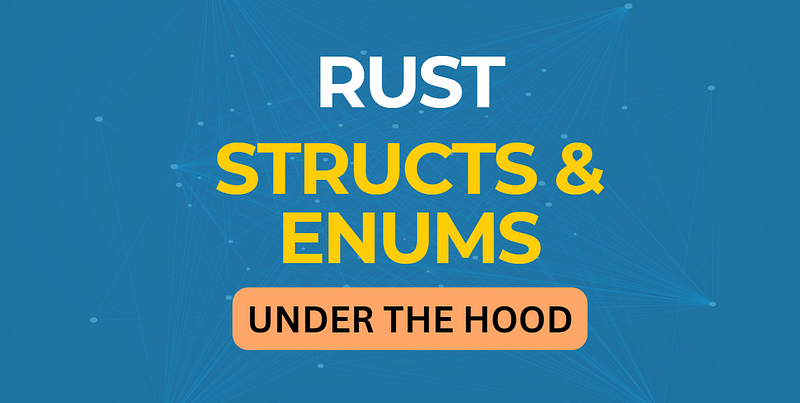
Understanding a bit about the inner mechanics and how structs and enums behave regarding memory allocation and performance provides deep and useful insights when learning the language.
Let’s dive into how Structs and Enums work behind the scenes and then jump to some practical examples and performance implications.
Structs in Rust
Structs, or structures, allow you to create custom data types by grouping together related values. They are useful for creating complex data types that represent real-world entities and their attributes.
Defining Structs
To define a struct, you use the struct
keyword followed by the name and the body defining the fields.
struct User {
username: String,
email: String,
sign_in_count: u64,
active: bool,
}
Instantiating Structs
To use a struct, you create an instance of it by specifying concrete values for each field.
let user1 = User {
email: String::from("someone@example.com"),
username: String::from("someusername123"),
active: true,
sign_in_count: 1,
};
Mutable Structs
If you want to change a value of a struct after it’s created, you must make the instance mutable.
let mut user1 = User {
email: String::from("someone@example.com"),
username: String::from("someusername123"),
active: true,
sign_in_count: 1,
};
user1.email = String::from("anotheremail@example.com");
Struct Update Syntax
Rust provides a syntax to create a new instance of a struct that uses most of an old instance’s values, with some changes.
let user2 = User {
email: String::from("another@example.com"),
..user1
};
Tuple Structs
Tuple structs have the fields indexed like tuples, which are useful when you want to give the whole tuple a name.
struct Color(i32, i32, i32);
let black = Color(0, 0, 0);
Enums in Rust
Enums, short for enumerations, allow you to define a type by enumerating its possible variants. Enums are particularly useful for defining types that can have a fixed set of values.
Defining Enums
You define an enum using the enum
keyword, followed by variants.
enum IpAddrKind {
V4,
V6,
}
let four = IpAddrKind::V4;
let six = IpAddrKind::V6;
Enums with Data
Enums can also store data. Each variant of an enum can have different types and amounts of associated data.
enum IpAddr {
V4(String),
V6(String),
}
let home = IpAddr::V4(String::from("127.0.0.1"));
Option Enum
Rust includes an Option<T>
enum to encode the very common scenario in which a value could be something or nothing.
enum Option<T> {
Some(T),
None,
}
let some_number = Some(5);
let some_string = Some("a string");
let absent_number: Option<i32> = None;
Use Cases and Comparison
- Structs are best used when you want to encapsulate related properties into one coherent type. For instance, if you’re going to represent a
Person
withname
,age
, andaddress
, a struct is an ideal choice. - Enums are more suited for types that can have a fixed set of variants. For example, defining a
NetworkError
type with variants likeTimeout
,Disconnected
, andUnknown
is a perfect use case for enums. - Use enums when you need pattern matching. Rust’s pattern matching with enums is powerful, allowing for clean and concise code when handling various cases.
- Use structs when you need to store and pass around related data that doesn’t have a fixed set of values. For instance, a
Rectangle
struct withwidth
andheight
fields is straightforward and intuitive.
Practical Example: Web Server
Imagine you’re building a simple web server in Rust. You could define an HttpRequest
struct to encapsulate request data and an HttpMethod
enum to represent the possible HTTP methods.
struct HttpRequest {
method: HttpMethod,
url: String,
headers: HashMap<String, String>,
body: String,
}
enum HttpMethod {
GET,
POST,
PUT,
DELETE,
}
let request = HttpRequest {
method: HttpMethod::GET,
url: String::from("/index.html"),
headers: HashMap::new(),
body: String::new(),
};
In this example, the HttpRequest
struct uses the HttpMethod
enum to specify the type of request. This design leverages the strengths of both structs and enums to create a more robust and expressive type system.
Structs and Memory Allocation
Structs in Rust are a way to group related data together. When a struct is instantiated, Rust allocates a contiguous block of memory for it, large enough to hold all its fields. The memory layout of a struct is determined primarily by its fields and their types.
Stack Allocation
By default, structs are allocated on the stack when they are created as local variables within a function. Stack allocation is fast because it involves merely moving the stack pointer. However, stack space is limited.
struct Point {
x: i32,
y: i32,
}
fn main() {
let point = Point { x: 10, y: 20 }; // Allocated on the stack
}
In this example, the Point
struct will be allocated on the stack, and the entire struct must fit within the stack frame of the main
function.
Heap Allocation
For larger structs or when you need the data to live beyond the current stack frame, you can allocate structs on the heap using a Box
.
fn main() {
let boxed_point = Box::new(Point { x: 10, y: 20 }); // Allocated on the heap
}
Heap allocation involves dynamically requesting memory at runtime. It is more flexible but also incurs a performance cost due to the allocation and deallocation overhead and potential cache misses.
Memory Layout
The memory layout of a struct is sequential, but padding may be added between fields to align the data according to the requirements of the underlying platform. This can affect the overall size of the struct.
Enums and Memory Allocation
Enums in Rust can have variants, each potentially holding different types and amounts of data. Rust needs to allocate enough memory to fit the largest variant. Additionally, Rust stores extra information to keep track of the current variant in use, usually as a “tag” or “discriminant”.
Fixed Memory Size
Regardless of which variant of an enum is currently in use, the memory size of the enum instance remains constant. This size is determined by the largest variant plus any space needed for the discriminant.
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(String),
}
fn main() {
let msg = Message::Write(String::from("hello")); // Allocates memory for the largest variant
}
In this case, the Message
enum must allocate enough memory to hold the largest variant, which is Write(String)
, plus the discriminant.
Tagged Unions
Enums in Rust can be thought of as “tagged unions.” A tagged union is a data structure that can hold any one of its variants at a time, with a “tag” indicating the current variant. This design impacts performance, as accessing the data requires reading the tag first, but it allows for more flexible and type-safe data structures.
Memory Optimization
For enums with variants of vastly different sizes, consider using Box
to store large data on the heap. This can reduce the overall size of the enum.
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(Box<String>), // Store large data on the heap
}
Performance Considerations
- Cache Locality: Structs, with their contiguous memory layout, tend to have better cache locality compared to enums with large variants. Better cache locality can lead to significant performance improvements, especially in data-intensive applications.
- Pattern Matching: Enums are often used with pattern matching, which introduces runtime checks to determine the variant. While Rust’s pattern matching is efficient, extensive use of complex patterns can impact performance.
- Memory Access: Accessing fields in a struct is generally faster than accessing data in an enum variant because structs don’t require reading a discriminant first. However, the difference is usually minimal unless in a performance-critical path.
- Allocation and Deallocation: Frequent allocations and deallocations, especially on the heap, can significantly impact performance. Structs and enums that are frequently created and destroyed may benefit from optimizations like using a pool allocator.
Optimization Strategies
Choosing Between Structs and Enums
When designing your data structures, carefully consider whether a struct or an enum is more appropriate:
- Use structs for data that is closely related and always present together, ensuring efficient memory layout and access patterns.
- Use enums for data that can vary significantly in type or size across different instances, leveraging Rust’s powerful pattern matching to handle various cases safely and succinctly.
Memory Usage Optimization
- For enums with large data variants, consider boxing the data to store it on the heap. This keeps the enum size small, especially when the enum is included in other structs or enums.
enum LargeEnum {
SmallVariant(u8),
LargeVariant(Box<[u8; 1024]>),
}
- When using structs with optional fields, consider using
Option<T>
to clearly indicate the presence or absence of data. This is particularly useful for avoiding the allocation of memory for fields that are often unused.
struct OptionalData {
mandatory: String,
optional: Option<String>,
}
Performance Optimization
- Initialization: Prefer initializing structs and enums with known values upfront using the struct update syntax or by directly setting the fields, which can be more efficient than multiple assignments.
let base_config = Config { port: 8080, ..Default::default() };
- Access Patterns: Analyze and optimize the access patterns to your data. Frequently accessed fields in a struct should be placed together to benefit from cache locality.
- Pattern Matching: While pattern matching with enums is idiomatic and clear, excessive or deeply nested pattern matching can lead to performance overhead. Keep pattern matching simple and consider refactoring very complex matches into simpler functions or using if let where appropriate.
match msg {
Message::Quit => handle_quit(),
Message::Move { x, y } => handle_move(x, y),
Message::Write(msg) => println!("{}", msg),
}
Best Practices
- Type Safety: Leverage Rust’s type system to ensure safety and correctness. Enums are particularly powerful for representing state and handling cases exhaustively, reducing runtime errors.
- Code Clarity: Opt for code clarity and maintainability, especially when choosing between structs and enums. A well-chosen data structure can make the code more intuitive and easier to work with.
- Memory Layout Considerations: Be mindful of the memory layout of your data structures. Rust’s default is to layout struct fields in the order they are defined, but you can use field reordering or explicit padding to optimize memory layout, though it’s rarely needed.
- Use of Derive: Utilize derive macros like
Clone
,Copy
,Debug
,PartialEq
, etc., to automatically implement common traits for your structs and enums, saving time and reducing boilerplate.
#[derive(Debug, Clone, Copy)]
struct Point {
x: i32,
y: i32,
}
🚀 Explore More by Luis Soares
📚 Learning Hub: Expand your knowledge in various tech domains, including Rust, Software Development, Cloud Computing, Cyber Security, Blockchain, and Linux, through my extensive resource collection:
- Hands-On Tutorials with GitHub Repos: Gain practical skills across different technologies with step-by-step tutorials, complemented by dedicated GitHub repositories. Access Tutorials
- In-Depth Guides & Articles: Deep dive into core concepts of Rust, Software Development, Cloud Computing, and more, with detailed guides and articles filled with practical examples. Read More
- E-Books Collection: Enhance your understanding of various tech fields with a series of free e-Books, including titles like “Mastering Rust Ownership” and “Application Security Guide” Download eBook
- Project Showcases: Discover a range of fully functional projects across different domains, such as an API Gateway, Blockchain Network, Cyber Security Tools, Cloud Services, and more. View Projects
- LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- Medium: Read my articles on Medium and give claps if you find them helpful. It motivates me to keep writing and sharing Rust content. Follow on Medium
- Personal Blog: Discover more on my personal blog, a hub for all my Rust-related content. Visit Blog
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- Twitter: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis.soares@linux.com
Senior Software Engineer | Cloud Engineer | SRE | Tech Lead | Rust | Golang | Java | ML AI & Statistics | Web3 & Blockchain