Rust Memory Layouts in Practice
Memory layout refers to how data is organized in memory, which affects performance, safety, and interoperability with other languages and…
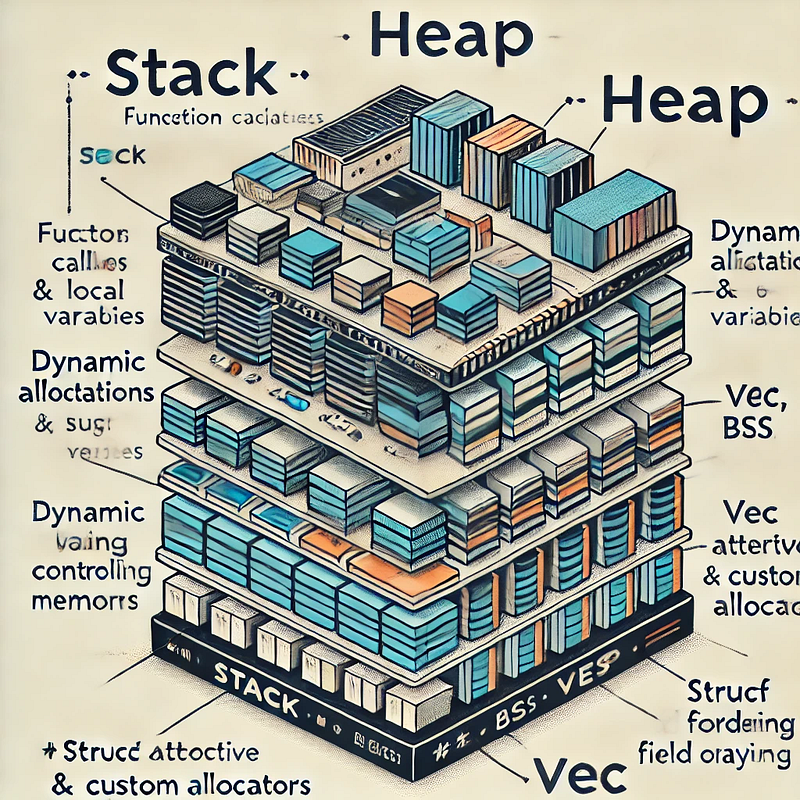
Memory layout refers to how data is organized in memory, which affects performance, safety, and interoperability with other languages and systems.
Memory Layout Basics
1. Stack vs. Heap
- Stack:
- The stack is used for storing local variables and function call information.
- It follows a Last-In-First-Out (LIFO) structure, which means the most recently allocated variables are the first to be deallocated.
- Stack allocation is fast but has limited size and is not suitable for dynamic or large allocations.
- Heap:
- The heap is used for dynamic memory allocation, where the size of data is not known at compile time or is too large to fit on the stack.
- Allocating and deallocating memory on the heap is slower compared to the stack.
- Rust provides
Box
,Rc
,Arc
, and other smart pointers to manage heap-allocated memory.
2. Data Segments
- Text Segment: Contains the compiled code of the program.
- Data Segment: Contains global and static variables that are initialized.
- BSS Segment: Contains uninitialized global and static variables.
Rust Memory Layouts
1. Structs and Enums
- Structs: The memory layout of structs in Rust is typically sequential. Each field is laid out in the order they are declared, with possible padding to satisfy alignment requirements.
struct MyStruct { a: u32, b: u64, c: u8, }
- In the above struct,
a
is placed at the start, followed by padding (if necessary), thenb
, and finallyc
with possible padding at the end to align the struct size to the largest field's alignment. - Enums: Enums in Rust are more complex, as they can contain different variants. The layout of an enum needs to accommodate the largest possible variant and includes a discriminant to identify which variant is currently active.
enum MyEnum { VariantA(u32), VariantB(u64), VariantC(u8), }
- The memory layout for
MyEnum
needs to be large enough to hold the largest variant (in this case,u64
) and a discriminant.
2. Arrays and Slices
- Arrays: Arrays are laid out sequentially in memory. Each element is placed next to the previous one, without any padding between them.
let arr: [i32; 3] = [1, 2, 3];
- Slices: Slices are dynamically sized views into arrays. They consist of a pointer to the data and a length. The data itself is stored sequentially.
let slice: &[i32] = &arr[1..3];
Alignment and Padding
Alignment refers to the requirement that data be placed at memory addresses that are multiples of their size. For example, a u32
typically needs to be aligned to a 4-byte boundary. If a type has stricter alignment requirements than the type preceding it, padding bytes are inserted to satisfy the alignment constraints.
Padding ensures that subsequent fields are correctly aligned. This is particularly relevant in structs, where fields of different sizes are placed together.
Layout Attributes
Rust provides attributes to control the memory layout of structs and enums:
repr(C)
: This attribute specifies that the memory layout should be compatible with C. This is essential for interoperability with C libraries and for predictable layout.
#[repr(C)] struct MyStruct { a: u32, b: u64, c: u8, }
repr(packed)
: This attribute removes padding between fields, which can be useful for certain binary formats but can lead to unaligned accesses.
#[repr(packed)] struct PackedStruct { a: u32, b: u64, c: u8, }
repr(align(N))
: This attribute forces the alignment of the struct to be at leastN
bytes.
#[repr(align(16))] struct AlignedStruct { a: u32, b: u64, }
Understanding Layout for Performance and Safety
- Performance: Proper understanding and control over memory layout can lead to significant performance improvements. Cache alignment, avoiding false sharing, and reducing padding can all contribute to more efficient memory access patterns.
- Safety: Ensuring correct memory layout is crucial for avoiding undefined behavior, especially when interfacing with other languages or systems. Attributes like
repr(C)
andrepr(packed)
help ensure that the layout matches the expectations of the environment.
Example: Analyzing Memory Layout
Here’s an example of how to analyze and understand the memory layout of a Rust struct:
use std::mem::{size_of, align_of};
#[repr(C)]
struct MyStruct {
a: u32,
b: u64,
c: u8,
}
fn main() {
println!("Size of MyStruct: {}", size_of::<MyStruct>());
println!("Alignment of MyStruct: {}", align_of::<MyStruct>());
println!("Offset of a: {}", offset_of!(MyStruct, a));
println!("Offset of b: {}", offset_of!(MyStruct, b));
println!("Offset of c: {}", offset_of!(MyStruct, c));
}
macro_rules! offset_of {
($ty:ty, $field:ident) => {
unsafe {
let base = std::ptr::null::<$ty>();
let field = &(*base).$field;
(field as *const _ as usize) - (base as *const _ as usize)
}
};
}
This example uses macros and standard library functions to print the size, alignment, and offsets of fields within a struct.
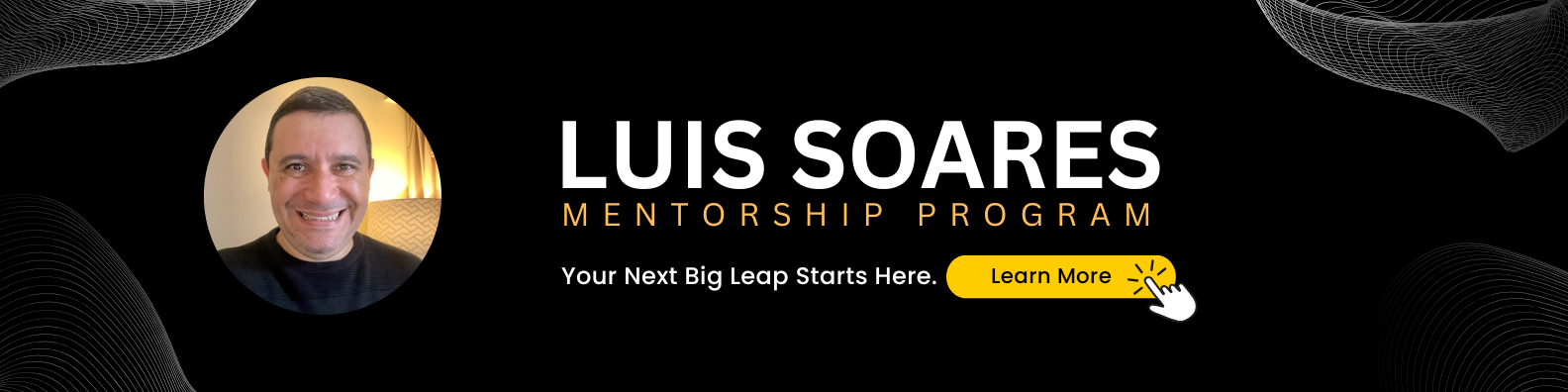
Advanced Memory Layout Techniques in Rust
In addition to basic memory layout management, Rust offers several advanced techniques and tools to fine-tune memory usage and layout, ensuring optimal performance and safety in complex scenarios.
1. Custom Allocators
Rust allows the use of custom allocators to manage memory allocation. This can be useful in scenarios where you need specialized memory management, such as in embedded systems, real-time systems, or high-performance applications.
Example of Custom Allocator:
use std::alloc::{GlobalAlloc, Layout, System};
struct MyAllocator;
unsafe impl GlobalAlloc for MyAllocator {
unsafe fn alloc(&self, layout: Layout) -> *mut u8 {
System.alloc(layout)
}
unsafe fn dealloc(&self, ptr: *mut u8, layout: Layout) {
System.dealloc(ptr, layout)
}
}
#[global_allocator]
static A: MyAllocator = MyAllocator;
fn main() {
let x = Box::new(42);
println!("Allocated value: {}", x);
}
In this example, MyAllocator
uses the system allocator, but you could implement your own allocation logic for more control.
2. Memory Pools and Arenas
Memory pools and arenas are techniques to manage memory efficiently by allocating large chunks of memory at once and sub-allocating from these chunks. This reduces the overhead of frequent small allocations and can improve cache performance.
Example of a Simple Memory Pool
use std::cell::RefCell;
struct MemoryPool {
pool: RefCell<Vec<u8>>,
}
impl MemoryPool {
fn new(size: usize) -> Self {
Self {
pool: RefCell::new(vec![0; size]),
}
}
fn allocate(&self, size: usize) -> Option<&mut [u8]> {
let mut pool = self.pool.borrow_mut();
if pool.len() >= size {
Some(&mut pool[..size])
} else {
None
}
}
}
fn main() {
let pool = MemoryPool::new(1024);
if let Some(memory) = pool.allocate(128) {
println!("Allocated 128 bytes from the pool");
} else {
println!("Allocation failed");
}
}
3. Layout Optimization
Optimizing the memory layout of data structures can have a significant impact on performance, particularly in terms of cache utilization and memory access patterns.
Field Reordering: Reordering fields in a struct to minimize padding can reduce the overall size of the struct and improve cache efficiency.
Example:
// Suboptimal ordering with padding
struct MyStruct {
a: u8,
b: u64,
c: u8,
}
// Optimal ordering to minimize padding
struct MyStructOptimized {
b: u64,
a: u8,
c: u8,
}
4. Using the #[repr]
Attribute
The #[repr]
attribute provides several options to control the memory layout of structs and enums:
#[repr(C)]
: Ensures compatibility with C, often used for FFI (Foreign Function Interface).#[repr(packed)]
: Removes padding between fields, but can lead to unaligned accesses.#[repr(align(N))]
: Forces a specific alignment.
Example:
#[repr(C)]
struct MyStructC {
a: u32,
b: u64,
}
#[repr(packed)]
struct MyStructPacked {
a: u32,
b: u64,
}
#[repr(align(16))]
struct MyStructAligned {
a: u32,
b: u64,
}
5. Interacting with Foreign Code
When interacting with C or other languages, controlling memory layout is crucial to ensure compatibility and prevent undefined behavior. Rust’s FFI (Foreign Function Interface) facilities combined with #[repr(C)]
help achieve this.
Example of FFI with C:
#[repr(C)]
struct MyCStruct {
a: i32,
b: f64,
}
extern "C" {
fn process_struct(s: *mut MyCStruct);
}
fn main() {
let mut s = MyCStruct { a: 42, b: 3.14 };
unsafe {
process_struct(&mut s);
}
}
6. Safe Abstractions and Zero-Cost Abstractions
Rust’s type system and ownership model provide powerful tools for creating safe abstractions without incurring runtime overhead. This is crucial for building complex systems where performance and safety are both critical.
Example of a Safe Abstraction:
struct SafeBox<T> {
value: T,
}
impl<T> SafeBox<T> {
fn new(value: T) -> Self {
Self { value }
}
fn get(&self) -> &T {
&self.value
}
fn get_mut(&mut self) -> &mut T {
&mut self.value
}
}
fn main() {
let mut safe_box = SafeBox::new(42);
println!("Value: {}", safe_box.get());
*safe_box.get_mut() = 43;
println!("Updated Value: {}", safe_box.get());
}
Whether you are working on embedded systems, high-performance applications, or interoperating with other languages, mastering memory layouts in Rust will help you build robust and efficient software.
🚀 Explore More by Luis Soares
📚 Learning Hub: Expand your knowledge in various tech domains, including Rust, Software Development, Cloud Computing, Cyber Security, Blockchain, and Linux, through my extensive resource collection:
- Hands-On Tutorials with GitHub Repos: Gain practical skills across different technologies with step-by-step tutorials, complemented by dedicated GitHub repositories. Access Tutorials
- In-Depth Guides & Articles: Deep dive into core concepts of Rust, Software Development, Cloud Computing, and more, with detailed guides and articles filled with practical examples. Read More
- E-Books Collection: Enhance your understanding of various tech fields with a series of e-Books, including titles like “Mastering Rust Ownership” and “Application Security Guide” Download eBook
- Project Showcases: Discover a range of fully functional projects across different domains, such as an API Gateway, Blockchain Network, Cyber Security Tools, Cloud Services, and more. View Projects
- LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- Medium: Read my articles on Medium and give claps if you find them helpful. It motivates me to keep writing and sharing Rust content. Follow on Medium
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- Twitter: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
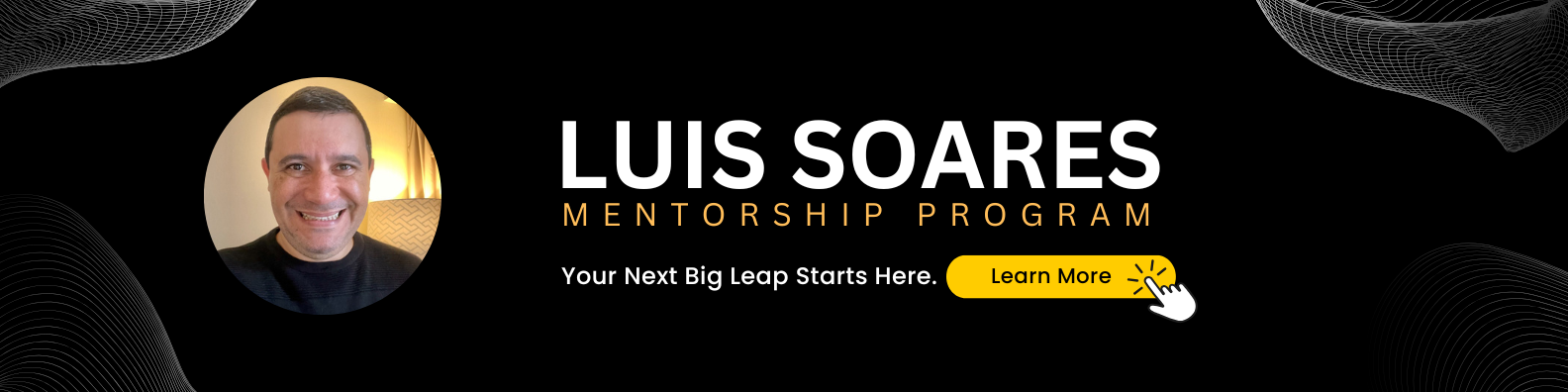
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis.soares@linux.com
Lead Software Engineer | Blockchain & ZKP Protocol Engineer | 🦀 Rust | Web3 | Solidity | Golang | Cryptography | Author