Rust: From Simple Functions to Advanced Abstractions
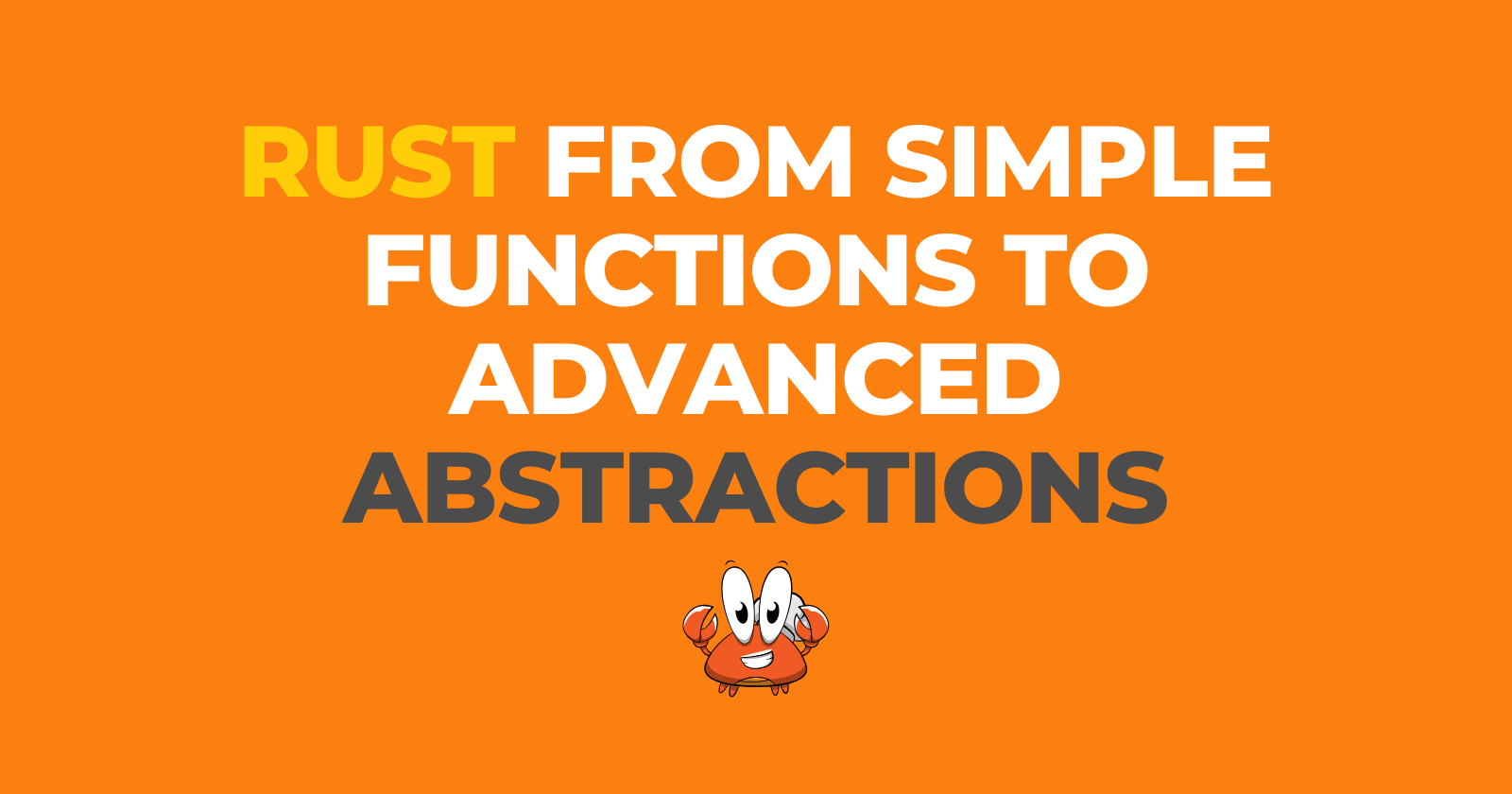
While many tutorials introduce isolated features, this article takes a holistic, practical approach: we’ll start with a simple example and iteratively enhance it to explore Rust’s powerful constructs.
By the end, you’ll clearly understand Rust’s core principles, from basic syntax to advanced abstractions like traits, lifetimes, macros, closures, and async programming.
Our journey begins with a basic calculator that performs simple arithmetic operations. We’ll refine and expand the calculator with each iteration, leveraging new Rust constructs to make it more flexible, reusable, and idiomatic.
Key Constructs We’ll Cover
- Functions and Basic Types
We’ll start with Rust fundamentals like defining functions, handling integer operations, and simple input/output. These basics form the foundation for our calculator. - Enums for Abstraction
Enums will represent operations like addition and subtraction, letting us group related functionality and reduce repetitive code. We’ll usematch
to handle branching logic. - Structs for Data Encapsulation
Structs allow us to bundle operands and operations into a single entity, making our code more organized and reusable. - Lifetimes for Safe Borrowing
Lifetimes ensure references remain valid while in use, preventing dangling references. We’ll apply them to borrowed data like strings in structs and functions, ensuring safety and memory efficiency. - Traits for Extensibility
Traits define shared behavior across types. ACalculator
trait will enable polymorphism, allowing different implementations without modifying existing code. - Generics for Flexibility
Generics will make our calculator work with any numeric type, like integers or floats, by abstracting over specific types while ensuring type safety through trait bounds. - Error Handling with
Result
We’ll improve robustness by handling errors like division by zero usingResult
and custom error types for clearer, safer error management. - Iterators for Data Processing
Iterators enable composable operations like summing sequences or filtering data. We’ll explore methods likemap
,filter
, andfold
to streamline calculations. - Closures for Dynamic Operations
Closures allow dynamic, user-defined operations. We’ll integrate closures to support inline calculations like|a, b| a.pow(b)
for flexibility. - Functional Programming Patterns
Using functional programming concepts, we’ll build higher-order functions, leverage closures, and chain iterators for declarative, efficient computation. - Macros for Reusable Code
Macros generate repetitive code and simplify patterns. We’ll usemacro_rules!
to automate operations like arithmetic function generation. - Smart Pointers for Dynamic Dispatch
UsingBox
anddyn
, we’ll introduce dynamic dispatch to handle runtime polymorphism, allowing flexible execution of different operation types. - Async Programming
To enable non-blocking calculations, we’ll use Rust’sasync
/await
syntax for concurrency, showcasing how to spawn tasks and integrate async workflows.
This journey will layer these concepts step by step, evolving our calculator into a powerful, flexible program while deepening your understanding of Rust.
1. The Foundations: Functions and Basic Types
A basic calculator in Rust starts with functions for each operation. For simplicity, we’ll begin with addition and subtraction.
fn add(a: i32, b: i32) -> i32 {
a + b
}
fn subtract(a: i32, b: i32) -> i32 {
a - b
}
fn main() {
let x = 10;
let y = 5;
println!("{} + {} = {}", x, y, add(x, y));
println!("{} - {} = {}", x, y, subtract(x, y));
}
This implementation is straightforward:
- We define two functions,
add
andsubtract
, each taking two integers and returning their sum or difference. - In
main
, we call these functions and print the results.
While this approach works for simple cases, adding more operations (e.g., multiplication, division) would lead to an explosion of functions. To improve, we can use enums to represent operations.
2. Introducing Enums to Represent Operations
Enums allow us to define a finite set of possible operations, such as Add
, Subtract
, Multiply
, and Divide
. This enables us to write a single function to handle all operations.
enum Operation {
Add,
Subtract,
Multiply,
Divide,
}
fn calculate(a: i32, b: i32, operation: Operation) -> i32 {
match operation {
Operation::Add => a + b,
Operation::Subtract => a - b,
Operation::Multiply => a * b,
Operation::Divide => {
if b != 0 {
a / b
} else {
eprintln!("Error: Division by zero is not allowed!");
0
}
}
}
}
fn main() {
let x = 20;
let y = 4;
println!("{} + {} = {}", x, y, calculate(x, y, Operation::Add));
println!("{} - {} = {}", x, y, calculate(x, y, Operation::Subtract));
println!("{} * {} = {}", x, y, calculate(x, y, Operation::Multiply));
println!("{} / {} = {}", x, y, calculate(x, y, Operation::Divide));
}
This implementation uses:
- An
Operation
enum to represent the type of operation. - A single
calculate
function that matches on theOperation
enum to perform the desired calculation.
By introducing the enum, we reduced redundancy and made it easier to add new operations. However, we can make this even cleaner by encapsulating the operands and operation in a struct.
3. Structuring the Data with Structs
By encapsulating the operands and the operation in a struct, we bundle related data into a single entity. This makes our code more readable and modular.
enum Operation {
Add,
Subtract,
Multiply,
Divide,
}
struct Calculation {
a: i32,
b: i32,
operation: Operation,
}
impl Calculation {
fn calculate(&self) -> i32 {
match self.operation {
Operation::Add => self.a + self.b,
Operation::Subtract => self.a - self.b,
Operation::Multiply => self.a * self.b,
Operation::Divide => {
if self.b != 0 {
self.a / self.b
} else {
eprintln!("Error: Division by zero!");
0
}
}
}
}
}
fn main() {
let calc1 = Calculation {
a: 15,
b: 3,
operation: Operation::Add,
};
let calc2 = Calculation {
a: 15,
b: 3,
operation: Operation::Divide,
};
println!("Result of calc1: {}", calc1.calculate());
println!("Result of calc2: {}", calc2.calculate());
}
In this example:
- The
Calculation
struct holds the operands (a
,b
) and the operation. - The
calculate
method performs the computation by matching onoperation
.
This organization makes it easier to manage calculations, but we can further enhance it by introducing lifetimes when dealing with borrowed data.
4. Adding Lifetimes for Borrowed Data
Suppose the operation type (e.g., "add"
, "subtract"
) is borrowed from user input. Rust requires us to use lifetimes to ensure that references remain valid while in use.
struct Calculation<'a> {
a: i32,
b: i32,
operation: &'a str,
}
impl<'a> Calculation<'a> {
fn calculate(&self) -> i32 {
match self.operation {
"add" => self.a + self.b,
"subtract" => self.a - self.b,
"multiply" => self.a * self.b,
"divide" => {
if self.b != 0 {
self.a / self.b
} else {
eprintln!("Error: Division by zero!");
0
}
}
_ => {
eprintln!("Error: Unsupported operation!");
0
}
}
}
}
fn main() {
let op = String::from("add");
let calc = Calculation {
a: 10,
b: 2,
operation: &op,
};
println!("Result: {}", calc.calculate());
}
Here:
- The
operation
field borrows a string, and the'a
lifetime ensures that the string outlives theCalculation
struct. - Lifetimes make the borrowing relationship explicit, preventing dangling references.
Next, let’s make our code extensible using traits.
5. Extensibility with Traits
We can introduce a Calculator
trait to define the behavior of any type of calculator, making our code more modular and reusable.
trait Calculator {
fn calculate(&self) -> i32;
}
struct AddCalculator {
a: i32,
b: i32,
}
struct MultiplyCalculator {
a: i32,
b: i32,
}
impl Calculator for AddCalculator {
fn calculate(&self) -> i32 {
self.a + self.b
}
}
impl Calculator for MultiplyCalculator {
fn calculate(&self) -> i32 {
self.a * self.b
}
}
fn main() {
let add_calc = AddCalculator { a: 7, b: 3 };
let mult_calc = MultiplyCalculator { a: 7, b: 3 };
println!("Addition result: {}", add_calc.calculate());
println!("Multiplication result: {}", mult_calc.calculate());
}
By using traits:
- We decouple behavior (
Calculator
) from data (AddCalculator
,MultiplyCalculator
). - New calculators can be added without modifying existing code.
Traits are powerful, but combining them with generics unlocks even more flexibility.
6. Generics for Flexible Calculations
Using generics, we can write a calculator that works with any numeric type.
use std::ops::{Add, Sub};
struct Calculation<T> {
a: T,
b: T,
}
impl<T> Calculation<T>
where
T: Add<Output = T> + Sub<Output = T> + Copy,
{
fn add(&self) -> T {
self.a + self.b
}
fn subtract(&self) -> T {
self.a - self.b
}
}
fn main() {
let int_calc = Calculation { a: 10, b: 5 };
let float_calc = Calculation { a: 3.5, b: 1.2 };
println!("Integer addition: {}", int_calc.add());
println!("Float subtraction: {}", float_calc.subtract());
}
Generics make the calculator versatile, supporting integers, floats, or any type that implements the required traits.
7. Closures for Dynamic Operations
Closures allow users to define custom operations dynamically. Here’s how we can use them in our calculator:
struct DynamicCalculation<'a> {
a: i32,
b: i32,
operation: Box<dyn Fn(i32, i32) -> i32 + 'a>,
}
fn main() {
let add = |x, y| x + y;
let multiply = |x, y| x * y;
let calc1 = DynamicCalculation {
a: 5,
b: 3,
operation: Box::new(add),
};
let calc2 = DynamicCalculation {
a: 5,
b: 3,
operation: Box::new(multiply),
};
println!("Result of calc1: {}", (calc1.operation)(calc1.a, calc1.b));
println!("Result of calc2: {}", (calc2.operation)(calc2.a, calc2.b));
}
This approach allows users to define operations inline, adding flexibility.
8. Functional Programming with Iterators
Functional programming patterns like iterators make calculations more composable.
fn main() {
let numbers = vec![1, 2, 3, 4];
let sum_of_squares: i32 = numbers.iter().map(|x| x * x).sum();
println!("Sum of squares: {}", sum_of_squares);
}
Here, chaining map
and sum
demonstrates how functional programming simplifies data processing.
9. Automating Patterns with Macros
Macros can reduce boilerplate by generating repetitive code.
macro_rules! operation {
($name:ident, $op:tt) => {
fn $name(a: i32, b: i32) -> i32 {
a $op b
}
};
}
operation!(add, +);
operation!(subtract, -);
fn main() {
println!("3 + 2 = {}", add(3, 2));
println!("3 - 2 = {}", subtract(3, 2));
}
This macro defines reusable arithmetic functions with minimal effort.
10. Async Programming for Concurrency
Asynchronous programming enables non-blocking calculations, perfect for heavy workloads.
use tokio::task;
struct AsyncCalculation {
a: i32,
b: i32,
}
impl AsyncCalculation {
async fn calculate(&self) -> i32 {
task::spawn_blocking(move || self.a + self.b).await.unwrap()
}
}
#[tokio::main]
async fn main() {
let calc = AsyncCalculation { a: 10, b: 5 };
println!("Result: {}", calc.calculate().await);
}
Async programming ensures responsiveness, even for long-running tasks.
We've deeply explored Rust's features by enhancing a simple step-by-step calculator. This journey demonstrates how Rust's constructs create robust, flexible, and safe programs, from basic types and functions to lifetimes, traits, generics, closures, macros, and async programming. Now, you’re equipped to take on more advanced Rust projects confidently!

🚀 Discover More Free Software Engineering Content! 🌟
If you enjoyed this post, be sure to explore my new software engineering blog, packed with 200+ in-depth articles, 🎥 explainer videos, 🎙️ a weekly software engineering podcast, 📚 books, 💻 hands-on tutorials with GitHub code, including:
🌟 Developing a Fully Functional API Gateway in Rust — Discover how to set up a robust and scalable gateway that stands as the frontline for your microservices.
🌟 Implementing a Network Traffic Analyzer — Ever wondered about the data packets zooming through your network? Unravel their mysteries with this deep dive into network analysis.
🌟Implementing a Blockchain in Rust — a step-by-step breakdown of implementing a basic blockchain in Rust, from the initial setup of the block structure, including unique identifiers and cryptographic hashes, to block creation, mining, and validation, laying the groundwork.
And much more!
✅ 200+ In-depth software engineering articles
🎥 Explainer Videos — Explore Videos
🎙️ A brand-new weekly Podcast on all things software engineering — Listen to the Podcast
📚 Access to my books — Check out the Books
💻 Hands-on Tutorials with GitHub code
📞 Book a Call
👉 Visit, explore, and subscribe for free to stay updated on all the latest: Home Page
LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- X: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis@luissoares.dev
Lead Software Engineer | Blockchain & ZKP Protocol Engineer | 🦀 Rust | Web3 | Solidity | Golang | Cryptography | Author