Mastering Generics in Rust: A Hands-on Guide
Generics allow us to define function signatures and data types with placeholder type instead of concrete types. This helps in writing more…
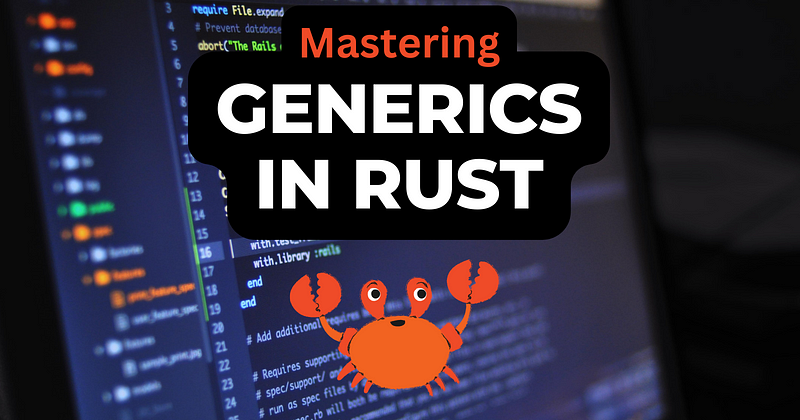
Generics allow us to define function signatures and data types with placeholder type instead of concrete types. This helps in writing more flexible and reusable code, without sacrificing the type safety and performance that Rust offers.
Basic Usage of Generics
Let’s start with a simple example to demonstrate the concept:
fn identity<T>(value: T) -> T {
value
}
let a = identity(5); // i32
let b = identity(5.5); // f64
let c = identity("Hello"); // &str
In the example above, the function identity
is defined with a generic type T
. When this function is called, Rust infers the type of T
based on the provided argument.
Generic Data Types
Rust allows you to define generic structs and enums as well. Here’s an example using a struct:
struct Point<T> {
x: T,
y: T,
}
let int_point = Point { x: 5, y: 10 }; // Point<i32>
let float_point = Point { x: 1.2, y: 3.4 }; // Point<f64>
Multiple Generic Types
You can use multiple generic types by separating them with commas:
struct Pair<T, U> {
first: T,
second: U,
}
let pair = Pair { first: "Hello", second: 42 }; // Pair<&str, i32>
Generics in Enum Definitions
Enums can also have generics:
enum Result<T, E> {
Ok(T),
Err(E),
}
The Result
type is a core part of Rust's error handling.
Generic Constraints & Traits
Sometimes, you want to restrict the types used with generics. Rust offers trait bounds to achieve this:
fn display<T: Display>(value: T) {
println!("{}", value);
}
Here, T: Display
means that the generic type T
must implement the Display
trait.
Implementing Methods on Generic Structs
When implementing methods for a generic struct, you can also specify trait bounds:
impl<T: Display + PartialOrd> Pair<T, T> {
fn max(&self) -> &T {
if self.first >= self.second {
&self.first
} else {
&self.second
}
}
}
In the example above, T
must implement both Display
and PartialOrd
traits.
The where Clause
While using generics with trait bounds, the syntax can sometimes get convoluted, especially with multiple bounds. Rust offers the where
clause for a cleaner approach:
fn some_function<T, U>(t: T, u: U)
where
T: Display + Clone,
U: Clone + Debug,
{
// function body
}
By using the where
clause, you can specify trait bounds in a more organized manner.
Lifetimes with Generics
In Rust, lifetimes specify how long references to data should remain valid. When working with generics, sometimes you need to specify lifetimes as well:
struct RefWrapper<'a, T> {
value: &'a T,
}
In the example above, 'a
is a lifetime parameter, and T
is a generic type parameter. This structure wraps a reference to a value of type T
with a given lifetime 'a
.
Associated Types with Generics
Traits can also define associated types. These allow for more flexible and concise trait definitions:
trait Iterator {
type Item;
fn next(&mut self) -> Option<Self::Item>;
}
In this example, Item
is an associated type. Implementors of this trait will specify what Item
should be, allowing for more varied and specific implementations.
Placeholder Types and the _ Operator
When you’re not interested in specifying or inferring a particular type for a generic, Rust allows you to use the _
placeholder:
let _numbers: Vec<_> = vec![1, 2, 3];
Here, Rust will infer the correct type for the vector’s items based on the provided values.
Advanced Trait Bounds with Generics
Beyond basic trait bounds, Rust allows for more advanced bounds that cater to specific needs:
Multiple Trait Bounds
You can specify multiple traits that a type must implement:
fn example<T: Display + Debug>(item: T) {
// function body
}
Here, T
must implement both the Display
and Debug
traits.
Trait Bounds on Structs and Enums
Generics can also be used in conjunction with trait bounds in struct and enum definitions:
struct GraphNode<T: Display> {
value: T,
// other fields
}
Conditional Implementation based on Trait
Sometimes, you may want to conditionally implement methods based on whether a type implements a specific trait:
impl<T> GraphNode<T> where T: Display {
fn display_value(&self) {
println!("{}", self.value);
}
}
Higher Ranked Trait Bounds (HRTB)
In some cases, you might encounter a situation where you need to express lifetimes about traits. This is where HRTB comes into play:
fn apply_fn<F>(f: F) where
F: for<'a> Fn(&'a str) -> &'a str,
{
// function body
}
The above function accepts a closure that can operate on a string slice of any lifetime.
Generic Defaults
Rust allows you to provide default types for generics, which is particularly useful when combined with traits:
trait Foo<T=usize> {
fn value(&self) -> T;
}
struct Bar;
impl Foo for Bar {
fn value(&self) -> usize {
42
}
}
In the above example, if the type for T
isn't supplied, it defaults to usize
.
Associated Constants in Traits
Along with associated types, Rust also allows you to define associated constants in traits:
trait Length {
const VALUE: usize;
}
struct Array;
impl Length for Array {
const VALUE: usize = 10;
}
With this, you can refer to Array::VALUE
to get the related constant.
Using Phantom Data with Generics
When working with generics, you might encounter situations where a type parameter is unused. This can lead to issues because Rust’s type system and ownership model rely on every type used. Here’s where PhantomData
comes into play:
use std::marker::PhantomData;
struct Wrapper<T> {
data: PhantomData<T>,
}
let _wrapper: Wrapper<f32> = Wrapper { data: PhantomData };
By using PhantomData
, you can tell Rust that Wrapper
might pretend to own a T
, even if it doesn't contain values of that type.
Coherence Rules with Generics
Rust’s orphan rule ensures a clear definition for trait implementations when generics are involved. This prevents conflicting trait implementations:
- Either the trait or the type should be defined in the local crate.
For instance, while you can implement your trait for a standard library type, you can’t implement a standard library trait for your type outside of the trait’s defining crate.
Variance with Generics
In Rust, variance defines how subtyping between more complex types relates to subtyping between their components. This is crucial when working with lifetimes. For instance:
&'a T
to&'b T
is covariant concerning'a
and'b
.
Understanding variance helps ensure you correctly use references and lifetimes with generics, keeping your Rust code safe and efficient.
Invariance
By default, Rust’s generics are invariant. This means, for example, even if T
is a subtype of U
, a Container<T>
won't be a subtype of Container<U>
. This default behaviour ensures maximum type safety in Rust's type system.
Generics and Dynamic Dispatch
While generics provide static dispatch, you may sometimes need dynamic dispatch, especially when the exact type is determined at runtime. In such cases, you can combine generics with Rust’s trait objects:
fn process_items<T: Trait + ?Sized>(items: &T) {
// function body
}
let items: &dyn Trait = &concrete_type;
process_items(items);
This uses dynamic dispatch, allowing for more flexibility at the cost of potential runtime overhead.
Read more articles about Rust in my Rust Programming Library!
Alright, we’ve covered quite a bit about generics in Rust, haven’t we? From the basics to some of the nitty-gritty details, this topic can initially seem a tad overwhelming.
But trust me, once you get the hang of it, you’ll see how these tools can help you craft elegant, efficient, and type-safe code. It’s like having a Swiss Army knife in your Rust toolkit! Remember, practice makes perfect.
So, dive in, write some code, and don’t be afraid to experiment.
Happy coding, and here’s to all the Rusty adventures ahead! 🦀
Read more articles about Rust in my Rust Programming Library!
Stay tuned, and happy coding!
Visit my Blog for more articles, news, and software engineering stuff!
Follow me on Medium, LinkedIn, and Twitter.
All the best,
CTO | Tech Lead | Senior Software Engineer | Cloud Solutions Architect | Rust 🦀 | Golang | Java | ML AI & Statistics | Web3 & Blockchain