Implementing Peer-to-Peer Data Exchange in Python
Peer-to-peer (P2P) networks are decentralized systems where nodes, or peers, communicate and exchange data directly without relying on a…
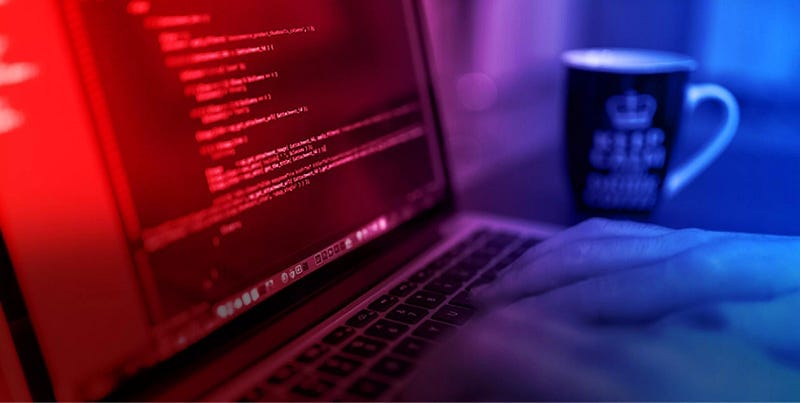
Peer-to-peer (P2P) networks are decentralized systems where nodes, or peers, communicate and exchange data directly without relying on a central server. This architecture has become increasingly popular due to its ability to distribute data efficiently and handle large amounts of traffic.
Python is an excellent choice for implementing P2P networks due to its simplicity, readability, and extensive library support. This article will walk you through implementing a basic P2P data exchange system in Python.
Prerequisites
To follow this tutorial, you should have a basic understanding of Python programming and experience working with sockets, threads, and the standard library. Familiarity with basic networking concepts is also helpful.
Step 1: Installing Required Libraries
We will use the ‘socket’ library for networking and ‘threading’ for concurrent connections. Both libraries are part of the Python standard library, so no additional installation is needed.
Step 2: Defining the Peer Class
The first step in implementing our P2P network is to define a Peer class that will represent each node in the network. This class will handle creating and managing connections with peers and exchanging data.
import socket
import threading
class Peer:
def __init__(self, host, port):
self.host = host
self.port = port
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.connections = []
Step 3: Creating Connections
We’ll now define a method within our Peer class to create connections with other peers. This method will establish a connection to another peer’s IP address and port and add the new connection to the connections
list.
def connect(self, peer_host, peer_port):
try:
connection = socket.create_connection((peer_host, peer_port))
self.connections.append(connection)
print(f"Connected to {peer_host}:{peer_port}")
except socket.error as e:
print(f"Failed to connect to {peer_host}:{peer_port}. Error: {e}")
Step 4: Listening for Incoming Connections
We also need to allow our peers to accept incoming connections from other nodes. This can be achieved by defining a ‘listen’ method within the Peer class that binds the socket to the host and port and starts listening for incoming connections.
def listen(self):
self.socket.bind((self.host, self.port))
self.socket.listen(10)
print(f"Listening for connections on {self.host}:{self.port}")
while True:
connection, address = self.socket.accept()
self.connections.append(connection)
print(f"Accepted connection from {address}")
Step 5: Implementing Data Exchange
Now we’ll define a method for exchanging data between peers. This method will iterate through all connections and send data to each peer.
def send_data(self, data):
for connection in self.connections:
try:
connection.sendall(data.encode())
except socket.error as e:
print(f"Failed to send data. Error: {e}")
Step 6: Creating a Multithreaded Peer
Since we want our Peer class to be able to both listen for incoming connections and send data simultaneously, we need to implement multithreading. We will add a method to start the listening thread when the Peer class is initialized.
def start(self):
listen_thread = threading.Thread(target=self.listen)
listen_thread.start()
Step 7: Testing the P2P Network
Now it’s time to test our P2P network by creating two Peer instances and exchanging data:
if __name__ == "__main__":
node1 = Peer("0.0.0.0", 8000)
node1.start()
node2 = Peer("0.0.0.0", 8001)
node2.start()
# Give some time for nodes to start listening
import time
time.sleep(2)
node2.connect("127.0.0.1", 8000)
time.sleep(1) # Allow connection to establish
node2.send_data("Hello from node2!")
Putting it all together
Here’s the full implementation:
import socket
import threading
class Peer:
def __init__(self, host, port):
self.host = host
self.port = port
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.connections = []
def connect(self, peer_host, peer_port):
connection = socket.create_connection((peer_host, peer_port))
self.connections.append(connection)
print(f"Connected to {peer_host}:{peer_port}")
def listen(self):
self.socket.bind((self.host, self.port))
self.socket.listen(10)
print(f"Listening for connections on {self.host}:{self.port}")
while True:
connection, address = self.socket.accept()
self.connections.append(connection)
print(f"Accepted connection from {address}")
threading.Thread(target=self.handle_client, args=(connection, address)).start()
def send_data(self, data):
for connection in self.connections:
try:
connection.sendall(data.encode())
except socket.error as e:
print(f"Failed to send data. Error: {e}")
self.connections.remove(connection)
def handle_client(self, connection, address):
while True:
try:
data = connection.recv(1024)
if not data:
break
print(f"Received data from {address}: {data.decode()}")
except socket.error:
break
print(f"Connection from {address} closed.")
self.connections.remove(connection)
connection.close()
def start(self):
listen_thread = threading.Thread(target=self.listen)
listen_thread.start()
# Example usage:
if __name__ == "__main__":
node1 = Peer("0.0.0.0", 8000)
node1.start()
node2 = Peer("0.0.0.0", 8001)
node2.start()
# Give some time for nodes to start listening
import time
time.sleep(2)
node2.connect("127.0.0.1", 8000)
time.sleep(1) # Allow connection to establish
node2.send_data("Hello from node2!")
Although a bare-bones example, this implementation can help you to get familiar with p2p systems from scratch.

🚀 Discover More Free Software Engineering Content! 🌟
If you enjoyed this post, be sure to explore my new software engineering blog, packed with 200+ in-depth articles, 🎥 explainer videos, 🎙️ a weekly software engineering podcast, 📚 books, 💻 hands-on tutorials with GitHub code, including:
🌟 Developing a Fully Functional API Gateway in Rust — Discover how to set up a robust and scalable gateway that stands as the frontline for your microservices.
🌟 Implementing a Network Traffic Analyzer — Ever wondered about the data packets zooming through your network? Unravel their mysteries with this deep dive into network analysis.
🌟Implementing a Blockchain in Rust — a step-by-step breakdown of implementing a basic blockchain in Rust, from the initial setup of the block structure, including unique identifiers and cryptographic hashes, to block creation, mining, and validation, laying the groundwork.
and much more!
✅ 200+ In-depth software engineering articles
🎥 Explainer Videos — Explore Videos
🎙️ A brand-new weekly Podcast on all things software engineering — Listen to the Podcast
📚 Access to my books — Check out the Books
💻 Hands-on Tutorials with GitHub code
📞 Book a Call
👉 Visit, explore, and subscribe for free to stay updated on all the latest: Home Page
LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- X: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis@luissoares.dev
Lead Software Engineer | Blockchain & ZKP Protocol Engineer | 🦀 Rust | Web3 | Solidity | Golang | Cryptography | Author