Handling multiple asynchronous operations easily with Rust ‘select!’
The select! macro is designed to manage multiple asynchronous operations, allowing a program to wait on several tasks simultaneously and…
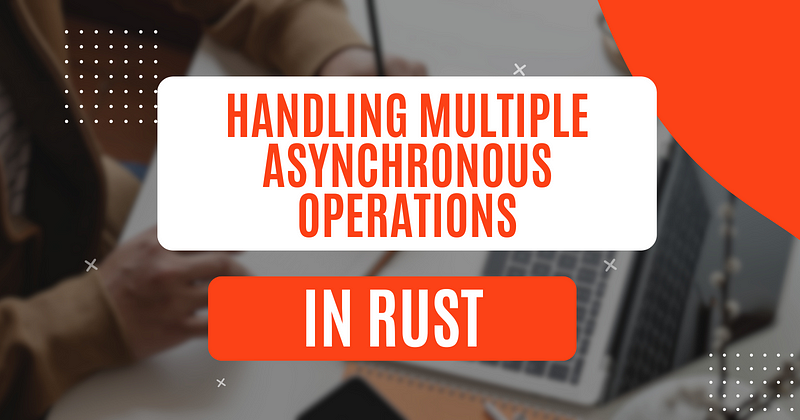
The select!
macro is designed to manage multiple asynchronous operations, allowing a program to wait on several tasks simultaneously and act on whichever completes first.
Understanding select!
The select!
macro in Rust is used to wait for multiple asynchronous tasks or events and handle the one that completes first. This is particularly useful in scenarios where you need to respond to multiple sources of input or events, such as in network servers or user interfaces.
Basic Syntax
The basic syntax of the select!
macro is as follows:
tokio::select! {
result1 = async_operation1() => {
// Handle the result of async_operation1
}
result2 = async_operation2() => {
// Handle the result of async_operation2
}
// Additional branches...
}
In this structure, each branch consists of a pattern (like result1 = async_operation1()
), followed by a block of code to execute if that operation completes first.
A Simple Example
Let’s start with a basic example:
async fn task_one() -> String {
tokio::time::sleep(tokio::time::Duration::from_secs(2)).await;
"Task one completed".to_string()
}
async fn task_two() -> String {
tokio::time::sleep(tokio::time::Duration::from_secs(1)).await;
"Task two completed".to_string()
}
#[tokio::main]
async fn main() {
tokio::select! {
result = task_one() => {
println!("First task completed: {}", result);
}
result = task_two() => {
println!("Second task completed: {}", result);
}
}
}
In this example, task_two()
will complete first because it has a shorter sleep duration. Therefore, the output will be "Second task completed: Task two completed".
Handling Cancellation
One of the key features of the select!
macro is its ability to handle cancellation gracefully. When one branch completes, all other branches are dropped. This means if you have an asynchronous operation that is no longer needed because another operation completed, it will be canceled automatically.
Here’s an example:
async fn long_running_task() {
tokio::time::sleep(tokio::time::Duration::from_secs(10)).await;
println!("Long running task completed");
}
async fn user_input() -> String {
tokio::time::sleep(tokio::time::Duration::from_secs(3)).await;
"User input received".to_string()
}
#[tokio::main]
async fn main() {
tokio::select! {
_ = long_running_task() => {
println!("The long running task finished first.");
}
input = user_input() => {
println!("Received user input: {}", input);
// Other operations are cancelled here
}
}
}
In this case, the user_input
function will likely complete first, and the long_running_task
will be canceled.
Combining with futures::future::Either
For more complex scenarios where you need to know which future completed without writing separate handlers, you can combine select!
with futures::future::Either
. This can be especially useful when dealing with a large number of futures.
use futures::future::Either;
#[tokio::main]
async fn main() {
let result = tokio::select! {
result = task_one() => Either::Left(result),
result = task_two() => Either::Right(result),
};
match result {
Either::Left(val) => println!("Task one completed with: {}", val),
Either::Right(val) => println!("Task two completed with: {}", val),
}
}
Advanced Usage
Adding a Default Case
The select!
macro also allows for a default case, which is executed if none of the other branches are ready:
tokio::select! {
result = async_operation() => {
// Handle the result
}
default => {
// This block executes if no other branches are ready
}
}
Combining with Loops
You can also use select!
inside loops for repeated operations:
#[tokio::main]
async fn main() {
let mut interval = tokio::time::interval(tokio::time::Duration::from_secs(1));
loop {
tokio::select! {
_ = interval.tick() => {
println!("Tick");
}
result = some_async_operation() => {
println!("Operation completed with: {}", result);
break;
}
}
}
}
In this loop, the program will print “Tick” every second until some_async_operation
completes.
Expanding the Use of select!
: Real-World Scenarios
As we delve deeper into the practical applications of Rust’s select!
macro, it becomes evident how versatile it is in real-world programming scenarios. Let's explore some of these situations and see how select!
enhances the efficiency and reliability of concurrent tasks.
Scenario: Timed Operations with Async I/O
A common use case in network programming is handling I/O operations with a timeout. Here’s how select!
can be applied:
async fn network_request() -> Result<String, &'static str> {
// Simulating a network request
tokio::time::sleep(tokio::time::Duration::from_secs(5)).await;
Ok("Network response".to_string())
}
#[tokio::main]
async fn main() {
let timeout = tokio::time::sleep(tokio::time::Duration::from_secs(3));
tokio::select! {
result = network_request() => {
match result {
Ok(response) => println!("Received response: {}", response),
Err(e) => println!("Network request failed: {}", e),
}
}
_ = timeout => {
println!("Request timed out");
}
}
}
In this scenario, the network request is simulated to take 5 seconds, but the timeout is set for 3 seconds. Hence, the timeout branch will be triggered, demonstrating how select!
can efficiently manage timed operations.
Scenario: Combining User Input with Asynchronous Events
Consider an application that needs to respond to user input while also processing asynchronous events. Here’s how select!
fits in:
async fn user_input() -> String {
// Function to receive user input
"User input".to_string()
}
async fn asynchronous_event() -> String {
// Function simulating an asynchronous event
tokio::time::sleep(tokio::time::Duration::from_secs(2)).await;
"Asynchronous event".to_string()
}
#[tokio::main]
async fn main() {
tokio::select! {
input = user_input() => {
println!("User input: {}", input);
}
event = asynchronous_event() => {
println!("Asynchronous event occurred: {}", event);
}
}
}
This example showcases how select!
can be used to handle user inputs and asynchronous events concurrently, enhancing the responsiveness of applications.
Scenario: Stream Processing
In applications that involve stream processing, like processing messages from a message queue, select!
can be invaluable:
async fn message_stream() -> String {
// Simulating a message from a stream
tokio::time::sleep(tokio::time::Duration::from_secs(1)).await;
"Message from stream".to_string()
}
async fn urgent_task() -> String {
// Simulating an urgent task
tokio::time::sleep(tokio::time::Duration::from_secs(5)).await;
"Urgent task completed".to_string()
}
#[tokio::main]
async fn main() {
tokio::select! {
message = message_stream() => {
println!("Received message: {}", message);
}
task = urgent_task() => {
println!("Urgent task finished: {}", task);
}
}
}
Here, select!
helps in prioritizing message processing from a stream while also keeping an eye on an urgent task.
Advanced Patterns: Nested select!
and Error Handling
For more complex scenarios, select!
can be nested, or combined with error handling mechanisms:
async fn operation_one() -> Result<String, &'static str> {
// Implementation...
Ok("Success from operation one")
}
async fn operation_two() -> Result<String, &'static str> {
// Implementation...
Ok("Success from operation two")
}
#[tokio::main]
async fn main() {
let result = tokio::select! {
result = operation_one() => result,
result = operation_two() => result,
};
match result {
Ok(message) => println!("Operation successful: {}", message),
Err(e) => println!("Operation failed: {}", e),
}
}
In this example, select!
is used to wait for multiple operations, and the result is processed with standard Rust error-handling techniques.
🚀 Explore a Wealth of Resources in Software Development and More by Luis Soares
📚 Learning Hub: Expand your knowledge in various tech domains, including Rust, Software Development, Cloud Computing, Cyber Security, Blockchain, and Linux, through my extensive resource collection:
- Hands-On Tutorials with GitHub Repos: Gain practical skills across different technologies with step-by-step tutorials, complemented by dedicated GitHub repositories. Access Tutorials
- In-Depth Guides & Articles: Deep dive into core concepts of Rust, Software Development, Cloud Computing, and more, with detailed guides and articles filled with practical examples. Read More
- E-Books Collection: Enhance your understanding of various tech fields with a series of free e-Books, including titles like “Mastering Rust Ownership” and “Application Security Guide” Download eBook
- Project Showcases: Discover a range of fully functional projects across different domains, such as an API Gateway, Blockchain Network, Cyber Security Tools, Cloud Services, and more. View Projects
- LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- Medium: Read my articles on Medium and give claps if you find them helpful. It motivates me to keep writing and sharing Rust content. Follow on Medium
- Personal Blog: Discover more on my personal blog, a hub for all my Rust-related content. Visit Blog
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- Twitter: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis.soares@linux.com
Senior Software Engineer | Cloud Engineer | SRE | Tech Lead | Rust | Golang | Java | ML AI & Statistics | Web3 & Blockchain