Functional Programming Patterns in Rust
Hey there!
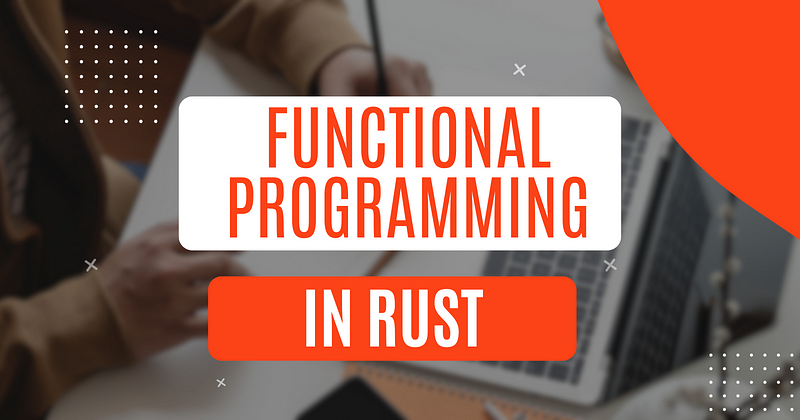
Hey there!
So, you’ve been tinkering with Rust, and maybe you’ve heard it’s not your average programming language. It’s got a reputation for being super meticulous about memory safety and concurrent programming, but that’s not all. Rust has some pretty neat tricks up its sleeve when it comes to functional programming patterns.
You might be thinking, “Functional programming, in Rust? But isn’t that more of a Haskell or Erlang thing?”
Well, yes and no. While Rust isn’t a pure functional language, it borrows a ton from that paradigm, giving you some powerful tools to write clean, maintainable code.
Let’s get our hands dirty with some practical examples and see how Rust can flex its functional muscles!
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It emphasizes the application of functions, often as first-class citizens, and the use of immutable data structures.
Fundamental Differences
Object-Oriented Programming (OOP):
- Stateful Objects: OOP is centered around objects that encapsulate state (data) and behavior (methods) together. The state of an object can change over time.
- Classes and Inheritance: It relies on the concept of classes as blueprints for objects and often uses inheritance to share and extend behavior between classes.
- Polymorphism: Objects of different classes can be treated as objects of a common superclass, especially if they share the same interface or base class.
- Imperative: OOP is generally imperative, focusing on how things should be done through the manipulation of object states.
Functional Programming (FP):
- Stateless Functions: FP focuses on stateless functions that operate on immutable data.
- First-Class Functions: Functions are first-class citizens and are used for abstraction, encapsulation, and composition.
- Recursion: Iterative processes are generally expressed through recursion.
- Declarative: FP is more declarative, specifying what should be done by expressing the logic of computation without describing its control flow.
Data and Behavior
In OOP, an object’s data and its related behavior are typically grouped together, which can be convenient for modeling real-world entities and relationships. OOP uses encapsulation to bundle the data and methods that operate on that data into one construct.
Conversely, FP aims to separate data from behavior. Data is typically represented in simple, immutable data structures, and behavior is represented with pure functions that operate on this data.
Mutability vs. Immutability
Mutability is a cornerstone of OOP. It allows object instances to change their state through methods. This mutability is natural for representing entities that need to change over time but can lead to complex state management and side effects.
FP, on the other hand, strives for immutability. Data structures are not allowed to change once created, which can lead to safer concurrent programming and functions that don’t cause side effects, making reasoning about and testing programs easier.
Inheritance vs. Composition
OOP heavily relies on inheritance, a mechanism to create a new class based on an existing class. This can lead to a tightly coupled hierarchy, which might become problematic to maintain.
FP prefers composition over inheritance. Functions are composed together to build more complex operations. This leads to loose coupling and easier maintainability.
Polymorphism
OOP employs polymorphism to invoke derived class methods through a base class reference, allowing for flexible and interchangeable objects.
In FP, polymorphism is achieved through higher-order functions and function types. Since functions are first-class citizens, they can be passed around as arguments and can be used to implement polymorphic behavior.
Concurrency
The immutable data structures in FP can make concurrent programming more straightforward. Since data cannot change, there are no locks or synchronization mechanisms needed.
OOP concurrency control typically involves managing locks and state to avoid issues like race conditions, which can be challenging.
Error Handling
OOP often manages errors and exceptions through try/catch mechanisms, which can interrupt the flow of the program and are often stateful.
FP handles errors as part of the normal flow, often using monads like Option
and Result
in Rust or Either
and Try
in Scala, which allow for error handling in a way that can be composed and treated like any other data.
Paradigm Use Cases
While FP is great for tasks that require high levels of abstraction, and are data-intensive with a lot of transformations, like in web services or data pipelines, OOP is often chosen for applications that closely model real-world objects and behaviors, like GUI applications or simulations.
In practice, many modern languages, including Rust, adopt a multi-paradigm approach, allowing developers to mix OOP and FP based on what best suits the problem at hand.
Embracing Functional Programming Patterns in Rust
Immutable Data Structures
Rust’s default for immutability promotes functional styles. Here's a simple example:
fn main() {
let x = 5; // x is immutable
// x = 6; // This line would cause a compile-time error
println!("The value of x is: {}", x);
}
To change the value, you need to explicitly use the mut
keyword, which is against the FP principle of immutability:
fn main() {
let mut x = 5;
x = 6; // Allowed because x is mutable
println!("The value of x is: {}", x);
}
Functional Error Handling
Rust uses Result
and Option
types for error handling, which is an application of the Maybe monad from functional programming:
fn divide(numerator: f64, denominator: f64) -> Option<f64> {
if denominator == 0.0 {
None
} else {
Some(numerator / denominator)
}
}
fn main() {
let result = divide(10.0, 2.0);
match result {
Some(quotient) => println!("Quotient: {}", quotient),
None => println!("Cannot divide by 0"),
}
}
Iterators and Lazy Evaluation
Rust's iterator pattern is a cornerstone of its functional approach, particularly with the use of lazy evaluation:
fn main() {
let numbers = vec![1, 2, 3, 4, 5];
let squares: Vec<_> = numbers.iter()
.map(|&x| x * x)
.filter(|&x| x > 10)
.collect();
println!("Squares greater than 10: {:?}", squares);
}
In this example, the .iter()
, .map()
, and .filter()
methods create an iterator pipeline that is only consumed and evaluated when .collect()
is called.
Concurrency Patterns
The following example demonstrates sharing immutable data between threads safely:
use std::sync::Arc;
use std::thread;
fn main() {
let data = Arc::new(vec![1, 2, 3]);
let mut handles = vec![];
for _ in 0..3 {
let data = Arc::clone(&data);
handles.push(thread::spawn(move || {
println!("{:?}", data);
}));
}
for handle in handles {
let _ = handle.join();
}
}
Here, Arc::clone
is used to provide thread-safe reference counting for our vector, allowing us to safely share read access with multiple threads.
Macros
Rust macros can be used to eliminate boilerplate and introduce new patterns. Here’s an example of a simple macro that mimics the map
function for Option
:
macro_rules! map_option {
($option:expr, $map_fn:expr) => {
match $option {
Some(value) => Some($map_fn(value)),
None => None,
}
};
}
fn main() {
let number = Some(3);
let squared_number = map_option!(number, |x| x * x);
println!("Squared number: {:?}", squared_number);
}
In this macro, map_option!
, we're abstracting away the pattern of applying a function to the Some
variant of an Option
.

Closures
Closures are anonymous functions that can capture their environment. They are extensively used in Rust, especially with iterators:
fn main() {
let factor = 2;
let multiplier = |x| x * factor; // `multiplier` is a closure capturing the `factor` from the environment.
let result: Vec<_> = (1..5).map(multiplier).collect();
println!("Results of multiplication: {:?}", result); // [2, 4, 6, 8]
}
In this example, multiplier
captures factor
from the surrounding environment, demonstrating how closures can encapsulate logic with context.
Recursion
Functional programming often relies on recursion as a mechanism for looping. Rust supports recursion, but you must be cautious about stack overflow. Tail recursion is not automatically optimized, but you can sometimes structure your code to take advantage of iterative optimizations:
fn factorial(n: u64) -> u64 {
fn inner_fact(n: u64, acc: u64) -> u64 {
if n == 0 {
acc
} else {
inner_fact(n - 1, acc * n) // recursive call
}
}
inner_fact(n, 1)
}
fn main() {
println!("Factorial of 5 is {}", factorial(5)); // Output: 120
}
In this recursive example, inner_fact
is a helper function that uses an accumulator, acc
, to hold the result as it recurses. This is a common functional pattern to handle state across recursive calls.
Pattern Matching
Pattern matching in Rust can be used in a variety of contexts and is particularly powerful in control flow and destructuring:
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(String),
ChangeColor(i32, i32, i32),
}
fn process_message(msg: Message) {
match msg {
Message::Quit => println!("Quit"),
Message::Move { x, y } => println!("Move to x: {}, y: {}", x, y),
Message::Write(text) => println!("Text message: {}", text),
Message::ChangeColor(r, g, b) => println!("Change color to red: {}, green: {}, blue: {}", r, g, b),
}
}
fn main() {
let messages = vec![
Message::Write(String::from("hello")),
Message::Move { x: 10, y: 30 },
Message::ChangeColor(0, 160, 255),
];
for msg in messages {
process_message(msg);
}
}
In process_message
, we're using match
to destructure the Message
enum and perform different actions based on its variant.
Monadic Combinators
Although Rust doesn’t have built-in monads like Haskell, you can use monadic combinators for Option
and Result
. For example, and_then
is similar to flatMap
in other languages:
fn square_root(x: f64) -> Option<f64> {
if x >= 0.0 { Some(x.sqrt()) } else { None }
}
fn reciprocal(x: f64) -> Option<f64> {
if x != 0.0 { Some(1.0 / x) } else { None }
}
fn main() {
let number = 4.0;
let result = square_root(number).and_then(reciprocal);
println!("The reciprocal of the square root of {} is {:?}", number, result);
}
In this snippet, and_then
is used to chain operations that may fail, where each function returns an Option
.
Advanced Iterators and Combinators
Rust’s iterators can be combined in powerful ways to perform complex transformations and computations in a clear and concise manner. Let’s take a look at a more advanced example that uses various combinators:
fn main() {
let numbers = vec![1, 2, 3, 4, 5];
let sum_of_squares: i32 = numbers.iter()
.map(|&x| x * x) // Maps each number to its square
.filter(|&x_square| x_square > 10) // Filters out squares <= 10
.fold(0, |acc, x_square| acc + x_square); // Sums up the remaining squares
println!("Sum of squares greater than 10: {}", sum_of_squares);
}
In this example, .iter()
, .map()
, .filter()
, and .fold()
are chained together to calculate the sum of squares greater than 10 in a single, succinct expression.
Option and Result Chaining
The Option
and Result
types can be used to write clean error handling without explicit match
statements. By using chaining, we can avoid deep nesting and create a pipeline of operations:
fn try_divide(dividend: f64, divisor: f64) -> Result<f64, &'static str> {
if divisor == 0.0 {
Err("Cannot divide by zero")
} else {
Ok(dividend / divisor)
}
}
fn main() {
let result = try_divide(10.0, 2.0)
.and_then(|quotient| try_divide(quotient, 0.0)) // Intentionally dividing by zero
.or_else(|err| {
println!("Encountered an error: {}", err);
try_divide(10.0, 2.0) // Providing an alternative operation
});
match result {
Ok(value) => println!("Result: {}", value),
Err(e) => println!("Error: {}", e),
}
}
This snippet shows how and_then
can be used for chaining operations that may produce a Result
, and or_else
provides an alternative in case of an error.
Lazy Evaluation with Iterators
Leveraging Rust’s iterator pattern, you can perform operations on potentially infinite sequences, thanks to lazy evaluation:
fn main() {
let fibonacci = std::iter::successors(Some((0, 1)), |&(prev, next)| Some((next, prev + next)))
.map(|(val, _)| val);
for num in fibonacci.take(10) { // Take only the first 10 elements
println!("{}", num);
}
}
Here, successors
creates an infinite iterator representing the Fibonacci sequence, but take
ensures that only the first 10 elements are computed and processed.
Type-Driven Development
In functional programming, the type system can often guide the development of functions. Rust’s powerful type system and type inference enable a style of programming where the types of the function inputs and outputs can determine the implementation:
// Define a generic function that takes an iterable of items that can be summed and returns the sum
fn sum_of_items<I, Item>(iterable: I) -> Item
where
I: IntoIterator<Item = Item>,
Item: std::iter::Sum,
{
iterable.into_iter().sum()
}
fn main() {
let numbers = vec![1, 2, 3, 4, 5];
let total: i32 = sum_of_items(numbers);
println!("Total sum is {}", total);
}
In this generic function, sum_of_items
, we don't need to know the specifics about the iterable or the item types, as long as they satisfy the constraints defined by the where
clause.
Wrap Up
We’ve just scratched the surface of functional programming patterns in Rust.
These patterns can help you write code that is not only safe and concurrent but also clean and modular. As you continue to write Rust code, remember that these functional patterns are tools in your toolbox.
They can be useful for handling data transformations, managing state, and writing code that’s less prone to bugs. The more you use these patterns, the more you’ll appreciate Rust’s ability to blend system-level control with high-level functional abstractions. Keep practicing and refining your approach to take full advantage of Rust’s functional capabilities.
Check out some interesting hands-on Rust articles!
🌟 Developing a Fully Functional API Gateway in Rust — Discover how to set up a robust and scalable gateway that stands as the frontline for your microservices.
🌟 Implementing a Network Traffic Analyzer — Ever wondered about the data packets zooming through your network? Unravel their mysteries with this deep dive into network analysis.
🌟 Building an Application Container in Rust — Join us in creating a lightweight, performant, and secure container from scratch! Docker’s got nothing on this.
🌟 Implementing a P2P Database in Rust: Today, we’re going to roll up our sleeves and get our hands dirty building a Peer-to-Peer (P2P) key-value database.
🌟 Building a Function-as-a-Service (FaaS) in Rust: If you’ve been exploring cloud computing, you’ve likely come across FaaS platforms like AWS Lambda or Google Cloud Functions. In this article, we’ll be creating our own simple FaaS platform using Rust.
🌟 Building an Event Broker in Rust: We’ll explore essential concepts such as topics, event production, consumption, and even real-time event subscriptions.

Read more articles about Rust in my Rust Programming Library!
Visit my Blog for more articles, news, and software engineering stuff!
Follow me on Medium, LinkedIn, and Twitter.
Leave a comment, and drop me a message!
All the best,
Luis Soares
CTO | Tech Lead | Senior Software Engineer | Cloud Solutions Architect | Rust 🦀 | Golang | Java | ML AI & Statistics | Web3 & Blockchain