Function Selectors in Solidity: Understanding and Working with Them
Introduction
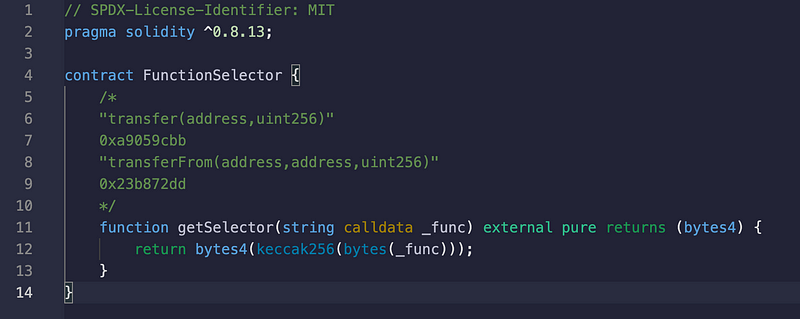
Introduction
Solidity, a statically typed programming language popularly known for writing smart contracts on Ethereum, employs several advanced features to ensure seamless and effective blockchain coding. One such feature is the 'Function Selector'. It is an essential part of the Ethereum function invocation process, contributing significantly to the efficient execution of smart contracts. Let's delve deeper into the concept to understand what function selectors are and how they work.
What is a Function Selector?
Function selectors are an integral part of the Application Binary Interface (ABI), the standard way to interact with contracts in the Ethereum ecosystem, both from outside the blockchain and for contract-to-contract interaction.
A function selector is a four-byte identifier determined through the Keccak (SHA-3) hash of the function's signature. The function signature is derived from the function name and the parenthesized list of parameter types. For instance, the function signature for a function named myFunction
that takes an address
and a uint256
would be myFunction(address,uint256)
.
The primary use of a function selector is to determine which function to call when multiple parts are present in a contract. In Ethereum, when a function call is made from an external source, it sends a data payload. The first four bytes of this payload contain the function selector, while the remaining bytes are reserved for any function arguments.
How Do Function Selectors Work?
Function selectors aim to encode the information about the function being called. When a contract is called, the EVM (Ethereum Virtual Machine) reads the first four bytes of the provided data to determine the function selector. The EVM uses this selector to match it with the correct function within the contract. If a match is found, the function is executed. If no match is found, the function call fails.
This function selection mechanism enables the contract interface to be highly flexible. New functions can be added without disturbing the existing ones, and function calls can specify precisely which function they intend to invoke.
Function Selectors in Practice
Let's consider an example to understand function selectors better:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.4;
contract Test {
function foo(uint256 _x, uint256 _y) public pure returns (uint256) {
return _x + _y;
}
function bar(address _addr) public pure returns (bool) {
return _addr == address(0);
}
}
In the above contract, we have two functions, foo
and bar
. The function signatures for these functions would be foo(uint256,uint256)
and bar(address)
, respectively. To create the function selector, we hash the signature using the Keccak-256 hash and take the first four bytes of the result.
In Solidity, we can get the function selector as follows:
bytes4 selector = bytes4(keccak256("foo(uint256,uint256)"));
This will give the selector for the function foo
. An external contract or account can now call the function foo
in the Test
contract by sending a transaction with data starting with this function selector, followed by the function arguments.
It's also worth noting that Solidity provides a shorthand for obtaining a function selector:
bytes4 selector = this.foo.selector;
The .selector
when appended to the function name, the keyword returns the function's selector.
Conclusion
Function selectors are an underpinning concept of the Ethereum Virtual Machine (EVM) and Solidity programming language. They encode the information about the function to be called, allowing the EVM to understand and execute the correct function. Although most Solidity developers may not deal with them directly, understanding function selectors and their role is crucial for deeply comprehending how Solidity and the EVM work.
Stay tuned, and happy coding!
Visit my Blog for more articles, news, and software engineering stuff!
Follow me on Medium, LinkedIn, and Twitter.
Check out my most recent book — Application Security: A Quick Reference to the Building Blocks of Secure Software.
All the best,
Luis Soares
CTO | Head of Engineering | Blockchain Engineer | Web3 | Cyber Security | Golang & eBPF Enthusiast
#blockchain #solidity #ethereum #smartcontracts #network #datastructures #communication #protocol #consensus #protocols #data #smartcontracts #web3 #security #privacy #confidentiality #cryptography #softwareengineering #softwaredevelopment #coding #software