Decentralized Networking with the libp2p Rust Library
Before we delve into the Rust crate, let’s understand what libp2p is and why it matters. Libp2p is a modular and extensible networking…
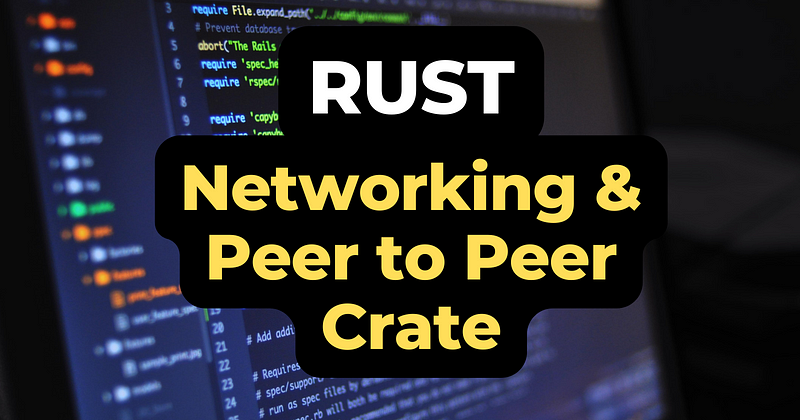
Before we delve into the Rust crate, let’s understand what libp2p is and why it matters. Libp2p is a modular and extensible networking stack designed to facilitate peer-to-peer communication. It was developed as part of the larger libp2p project, which aims to create a set of interoperable networking modules for various use cases.
Libp2p was built with decentralization in mind, making it an ideal choice for applications such as blockchain networks, distributed file systems, and secure messaging platforms. It’s protocol-agnostic, which means it can support various communication protocols, making it adaptable to different scenarios.
The libp2p Rust Crate
The libp2p Rust crate is the Rust implementation of libp2p, bringing its powerful features to the Rust programming language. Rust is known for its focus on memory safety and performance, making it a fantastic choice for building robust and efficient networking applications. The libp2p Rust crate provides Rust developers a flexible and efficient toolkit for building peer-to-peer applications.
Components of the libp2p Rust Crate
- Transport: The transport layer sends and receives data between peers. The libp2p Rust crate supports various transport protocols, such as TCP, WebSockets, and QUIC. This flexibility allows developers to choose the transport protocol that best suits their application’s requirements.
- Peer Discovery: Discovering other peers on the network is a crucial aspect of peer-to-peer communication. The libp2p Rust crate offers multiple peer discovery mechanisms, including mDNS, Kademlia DHT (Distributed Hash Table), and custom discovery methods.
- Connection Upgrading: Libp2p allows for secure and efficient communication by upgrading connections to support different protocols. This ensures that the most suitable protocol is used for each communication session.
- Multiplexing: Multiplexing allows multiple data streams to be transmitted over a single connection simultaneously. The libp2p Rust crate supports various multiplexing protocols like Mplex and yamux.
- Security: Security is paramount in peer-to-peer networks. Libp2p offers built-in cryptographic authentication and encryption support to ensure data privacy and integrity.
- Protocols: Libp2p is protocol-agnostic, enabling developers to define custom application protocols. It also supports popular protocols like DNS, BitSwap, and QUIC.
Use Cases of the libp2p Rust Crate
- Blockchain Networks: Many blockchain networks rely on decentralized peer-to-peer communication. The libp2p Rust crate’s versatility and robustness make it an excellent choice for building blockchain nodes and enabling efficient communication between them.
- Distributed File Systems: Building distributed file systems like IPFS (InterPlanetary File System) becomes more accessible with libp2p. The libp2p Rust crate’s modular architecture allows developers to create efficient file-sharing networks.
- Secure Messaging Platforms: Privacy-focused messaging apps can benefit from libp2p’s secure and encrypted communication capabilities. It enables users to communicate directly, reducing the need for central servers.
- IoT Devices: The Internet of Things (IoT) demands efficient and decentralized communication between devices. Libp2p’s peer discovery and transport options make it suitable for IoT applications.
- Decentralized Applications (DApps): DApps often rely on peer-to-peer communication for various functionalities. Libp2p simplifies the development of DApps by providing a robust networking layer.
Hands-on Examples
1. Creating a TCP Transport and Establishing a Connection
In this example, we’ll create a simple Rust program that uses libp2p to establish a TCP connection between two peers.
use async_std::task;
use libp2p::{
core::identity,
noise::{Keypair, NoiseConfig, X25519Spec},
tcp::TcpConfig,
yamux::YamuxConfig,
core::upgrade::read_one,
identity::KeypairIdentity,
};
use futures::prelude::*;
use std::error::Error;
#[tokio::main]
async fn main() -> Result<(), Box<dyn Error>> {
// Generate local peer keypair
let local_keys = Keypair::<X25519Spec>::new().into_authentic(&identity::Keypair::<identity::ed25519::Ed25519KeyPair>::new().unwrap())?;
// Create TCP transport
let transport = TcpConfig::new();
// Create Noise configuration for secure communication
let noise = NoiseConfig::xx(local_keys).into_authenticated();
// Create Yamux multiplexer configuration
let yamux = YamuxConfig::default();
// Build the libp2p stack
let mut swarm = {
let (peer_id, transport) = transport.upgrade(libp2p::core::upgrade::Version::V1).await?;
libp2p::core::Swarm::new(
transport
.upgrade(libp2p::core::upgrade::Version::V1)
.authenticate(noise)
.multiplex(yamux)
.boxed(),
peer_id.clone(),
libp2p::core::topology::MemoryTopology::empty(),
peer_id.clone(),
Default::default(),
)
};
// Listen on a random TCP port
let listen_address = "/ip4/0.0.0.0/tcp/0".parse()?;
swarm.listen_on(listen_address)?;
println!("Local peer id: {:?}", swarm.local_peer_id());
// Main event loop
loop {
match swarm.select_next_some().await {
libp2p::core::either::EitherOutput::First((peer_id, connection)) => {
println!("Connected to {:?}", peer_id);
tokio::spawn(handle_connection(connection));
}
_ => {}
}
}
}
async fn handle_connection(mut connection: libp2p::core::upgrade::read_one::ReadOne<libp2p::tcp::TcpStream>) -> Result<(), Box<dyn Error>> {
let mut buf = Vec::new();
connection.read_to_end(&mut buf).await?;
let received_message = String::from_utf8_lossy(&buf);
println!("Received message: {}", received_message);
Ok(())
}
This example creates a simple libp2p program that listens on a random TCP port and establishes connections with other peers.
2. Kademlia DHT for Peer Routing
In this example, we’ll use libp2p’s Kademlia Distributed Hash Table (DHT) to discover peers and route messages between them.
use async_std::task;
use libp2p::{
core::identity,
noise::{Keypair, NoiseConfig, X25519Spec},
tcp::TcpConfig,
yamux::YamuxConfig,
core::upgrade::read_one,
identity::KeypairIdentity,
mdns::{Mdns, MdnsEvent},
kad::{record::store::MemoryStore, Kademlia},
multiaddr::{Protocol, Multiaddr},
};
use futures::prelude::*;
use std::error::Error;
#[tokio::main]
async fn main() -> Result<(), Box<dyn Error>> {
// Generate local peer keypair
let local_keys = Keypair::<X25519Spec>::new().into_authentic(&identity::Keypair::<identity::ed25519::Ed25519KeyPair>::new().unwrap())?;
// Create TCP transport
let transport = TcpConfig::new();
// Create Noise configuration for secure communication
let noise = NoiseConfig::xx(local_keys).into_authenticated();
// Create Yamux multiplexer configuration
let yamux = YamuxConfig::default();
// Build the libp2p stack
let mut swarm = {
let (peer_id, transport) = transport.upgrade(libp2p::core::upgrade::Version::V1).await?;
libp2p::core::Swarm::new(
transport
.upgrade(libp2p::core::upgrade::Version::V1)
.authenticate(noise)
.multiplex(yamux)
.boxed(),
peer_id.clone(),
libp2p::core::topology::MemoryTopology::empty(),
peer_id.clone(),
Default::default(),
)
};
// Listen on a random TCP port
let listen_address = "/ip4/0.0.0.0/tcp/0".parse()?;
swarm.listen_on(listen_address)?;
println!("Local peer id: {:?}", swarm.local_peer_id());
// Create Kademlia DHT
let local_peer_id = swarm.local_peer_id().clone();
let store = MemoryStore::new(local_peer_id.clone());
let mut kademlia = Kademlia::new(local_peer_id.clone(), store);
// Start the mDNS service to discover peers
let mdns = Mdns::new()?;
swarm.behaviour_mut().dialer_mut().add_discovered_node(local_peer_id.clone());
swarm.behaviour_mut().dialer_mut().add_unreachable_node(local_peer_id.clone());
// Main event loop
loop {
match swarm.select_next_some().await {
libp2p::core::either::EitherOutput::First((peer_id, connection)) => {
println!("Connected to {:?}", peer_id);
tokio::spawn(handle_connection(connection));
}
libp2p::core::either::EitherOutput::Second(MdnsEvent::Discovered(peers)) => {
for (peer_id, _addr) in peers {
// Add discovered peers to Kademlia DHT
kademlia.add_address(&peer_id, Protocol::P2p(peer_id.clone().into()));
}
}
_ => {}
}
}
}
async fn handle_connection(mut connection: libp2p::core::upgrade::read_one::ReadOne<libp2p::tcp::TcpStream>) -> Result<(), Box<dyn Error>> {
let mut buf = Vec::new();
connection.read_to_end(&mut buf).await?;
let received_message = String::from_utf8_lossy(&buf);
println!("Received message: {}", received_message);
Ok(())
}
This example combines Kademlia DHT with mDNS for peer discovery and routing messages between peers.
These examples provide a basic understanding of how to use libp2p in Rust to establish connections and implement Kademlia DHT for peer routing. You can build upon these examples to create more complex peer-to-peer applications.
🚀 Explore a Wealth of Resources in Software Development and More by Luis Soares
📚 Learning Hub: Expand your knowledge in various tech domains, including Rust, Software Development, Cloud Computing, Cyber Security, Blockchain, and Linux, through my extensive resource collection:
- Hands-On Tutorials with GitHub Repos: Gain practical skills across different technologies with step-by-step tutorials, complemented by dedicated GitHub repositories. Access Tutorials
- In-Depth Guides & Articles: Deep dive into core concepts of Rust, Software Development, Cloud Computing, and more, with detailed guides and articles filled with practical examples. Read More
- E-Books Collection: Enhance your understanding of various tech fields with a series of free e-Books, including titles like “Mastering Rust Ownership” and “Application Security Guide” Download eBook
- Project Showcases: Discover a range of fully functional projects across different domains, such as an API Gateway, Blockchain Network, Cyber Security Tools, Cloud Services, and more. View Projects
- LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- Medium: Read my articles on Medium and give claps if you find them helpful. It motivates me to keep writing and sharing Rust content. Follow on Medium
- Personal Blog: Discover more on my personal blog, a hub for all my Rust-related content. Visit Blog
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- Twitter: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis.soares@linux.com
Senior Software Engineer | Cloud Engineer | SRE | Tech Lead | Rust | Golang | Java | ML AI & Statistics | Web3 & Blockchain