A Binary Disassembler in Rust
Disassemblers are tools that translate machine code back into assembly language, providing insights into the inner workings of compiled…
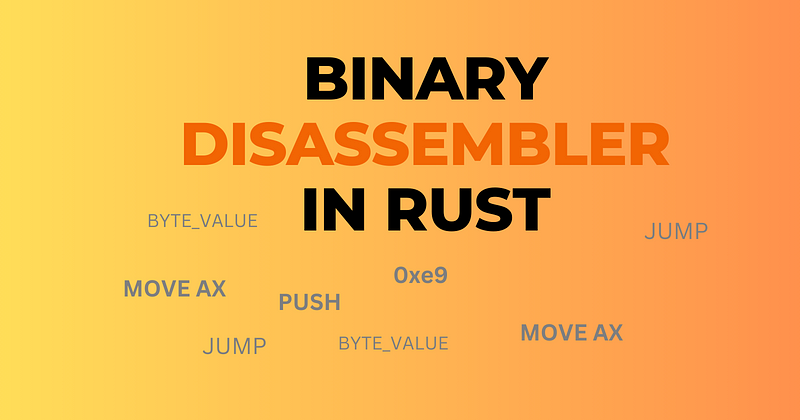
Disassemblers are tools that translate machine code back into assembly language, providing insights into the inner workings of compiled programs.
Today, let’s delve into the construction of a disassembler in Rust, leveraging the capabilities of the Capstone disassembly framework through the capstone
crate.
Key Concepts
Disassembly
Disassembly is the process of converting machine code, the binary instructions that a computer directly executes, back into a human-readable assembly language. This process is crucial for reverse engineering, debugging, and understanding the low-level operations of software.
Assembly Language
Assembly language is a low-level programming language that closely represents the machine code instructions of a computer architecture. It provides mnemonics for operations (e.g., MOV
, ADD
, JMP
) and is specific to a particular processor architecture (e.g., x86, ARM).
Capstone Disassembly Framework
Capstone is a lightweight, multi-platform, multi-architecture disassembly framework. It offers bindings for various programming languages, including Rust, making it accessible for a wide range of applications.
Capstone supports numerous architectures, such as x86, ARM, MIPS, and PowerPC, and provides detailed disassembly information, making it a versatile tool for building disassemblers.
Background on Capstone
Developed with reverse engineering and binary analysis in mind, Capstone provides a rich set of features:
- Multi-architecture Support: Capstone can disassemble binary code for various architectures, making it highly versatile.
- Detailed Instruction Decoding: It provides extensive details about disassembled instructions, including operands, instruction groups, and CPU flags affected.
- Flexible Disassembly Modes: Capstone supports different disassembly modes, such as 16-bit, 32-bit, and 64-bit, accommodating various application needs.
- Customizable Syntax: The output assembly syntax (e.g., Intel, AT&T) can be tailored to user preferences.
Core Components
- Engine: The Capstone engine is the primary component responsible for the disassembly process. It is initialized with specific parameters such as the target CPU architecture and mode (e.g., 32-bit, 64-bit). The engine is highly configurable, allowing users to set options like syntax (e.g., Intel, AT&T), detail level, and custom instruction boundaries.
- Decoders: For each supported architecture (e.g., x86, ARM, MIPS), Capstone has a dedicated decoder. These decoders are responsible for parsing the binary machine code and translating it into an intermediate representation (IR). The IR abstracts the machine code into a form that Capstone’s engine can analyze and process further.
- Instruction Set: Capstone includes comprehensive instruction sets for each supported architecture. These instruction sets define the properties and behaviors of each instruction, including operands, instruction groups (e.g., jump, call, return), and affected flags. This information is crucial for accurately disassembling and interpreting machine code.
- Syntax Converters: Capstone supports multiple assembly syntaxes, such as Intel and AT&T for x86 architecture. Syntax converters take the disassembled instructions from the IR and format them according to the selected syntax, making the output customizable to the user’s preferences.
- Detail API: When detail mode is enabled, Capstone provides extensive information about each disassembled instruction, including operands, instruction groups, CPU flags affected, and more. This detailed information is gathered from the instruction’s metadata and the architecture-specific details encoded in the machine code.
At a low level, Capstone performs numerous binary and bitwise operations to decode machine code. It handles various encoding schemes, opcode tables, and CPU-specific quirks. The framework meticulously manages endianness, instruction prefixes (e.g., operand-size override, address-size override in x86), and conditional code execution (e.g., ARM’s condition flags) to ensure accurate disassembly.
Building a Simple Disassembler
Step 1: Initialize Your Rust Project
Create a New Project: Open a terminal and run the following command to create a new Rust project:
cargo new rust_disassembler
Step 2: Add Dependencies
Open the Cargo.toml
file located in your project directory and add the capstone
and clap
crates to your dependencies section:
[dependencies]
capstone = "0.10.0" # Use the latest version available on crates.io
clap = "3.1.18" # Adjust to the latest version as needed
Step 3: Set Up Command-Line Argument Parsing
Open the src/main.rs
file and set up the clap
crate to handle command-line arguments. For now, include an argument for the binary file path:
use clap::{App, Arg};
fn main() {
let matches = Command::new("Rust Disassembler")
.version("1.0")
.author("Your Name <your_email@example.com>")
.about("Disassembles binary files")
.arg(Arg::new("FILE")
.help("Sets the input file to use")
.required(true)
.index(1))
.get_matches();
let file_path = matches.get_one::<String>("FILE").expect("FILE is required");
let binary_data = read_binary_file(file_path).expect("Failed to read binary file");
// Placeholder for further steps
}
Step 4: Implement Binary File Reading
Extend main.rs
to include a function that reads the specified binary file into a byte vector:
use std::fs::File;
use std::io::{self, Read};
use std::path::Path;
fn read_binary_file<P: AsRef<Path>>(path: P) -> io::Result<Vec<u8>> {
let mut file = File::open(path)?;
let mut buffer = Vec::new();
file.read_to_end(&mut buffer)?;
Ok(buffer)
}
Step 5: Initialize Capstone for Disassembly
Import Capstone in main.rs
and set up the disassembly engine. This example uses the x86_64 architecture, but you can modify it for other architectures:
use capstone::prelude::*;
fn disassemble(code: &[u8]) {
let cs = Capstone::new()
.x86()
.mode(arch::x86::ArchMode::Mode64)
.syntax(arch::x86::ArchSyntax::Intel)
.detail(true) // Enable detailed disassembly
.build()
.expect("Failed to create Capstone disassembler");
let insns = cs.disasm_all(code, 0x1000).expect("Failed to disassemble"); // Assuming 0x1000 as the base address
for insn in insns.iter() {
println!("0x{:x}: {:6} {}", insn.address(), insn.mnemonic().unwrap_or(""), insn.op_str().unwrap_or(""));
// Placeholder for detailed output
}
}
In the main
function, read the binary file and pass its contents to the disassemble
function:
// Inside main()
let binary_data = read_binary_file(file_path).expect("Failed to read binary file");
disassemble(&binary_data);
Step 6: Enhance Output with Capstone’s Detail API
Modify the disassemble
function to include detailed information about each instruction, such as registers read and written:
// Inside the disassemble() function, after printing basic instruction info
if let Ok(detail) = cs.insn_detail(&insn) {
println!(" Bytes: {:?}", insn.bytes());
println!(" Read regs: {:?}", detail.regs_read());
println!(" Write regs: {:?}", detail.regs_write());
// Expand this section to include more details as needed
}
Step 7: Compile and Run Your Disassembler
Compile your project using Cargo:
cargo build
Execute your disassembler, providing the path to a binary file as an argument:
cargo run -- /path/to/your/binary/file
Advanced Usage
Handling Multiple Architectures
Capstone’s architecture-agnostic design allows for easy extension to other CPU architectures. You can abstract the initialization process into a function that takes an architecture parameter and returns a configured Capstone instance.
Analyzing Instruction Details
Capstone’s detailed mode enables access to a wealth of information about each instruction, such as operands, instruction groups, and affected CPU flags. Utilize the insn.detail()
method to explore this data.
Custom Syntax and Modes
Adjust the disassembly syntax and mode to fit your needs. Capstone supports various syntax styles (e.g., Intel, AT&T) and modes (e.g., 16-bit, 32-bit) that can be configured during the initialization of the Capstone instance.
You can read all about the Capstone Disassembly Framework on its documentation.
🚀 Explore a Wealth of Resources in Software Development and More by Luis Soares
📚 Learning Hub: Expand your knowledge in various tech domains, including Rust, Software Development, Cloud Computing, Cyber Security, Blockchain, and Linux, through my extensive resource collection:
- Hands-On Tutorials with GitHub Repos: Gain practical skills across different technologies with step-by-step tutorials, complemented by dedicated GitHub repositories. Access Tutorials
- In-Depth Guides & Articles: Deep dive into core concepts of Rust, Software Development, Cloud Computing, and more, with detailed guides and articles filled with practical examples. Read More
- E-Books Collection: Enhance your understanding of various tech fields with a series of free e-Books, including titles like “Mastering Rust Ownership” and “Application Security Guide” Download eBook
- Project Showcases: Discover a range of fully functional projects across different domains, such as an API Gateway, Blockchain Network, Cyber Security Tools, Cloud Services, and more. View Projects
- LinkedIn Newsletter: Stay ahead in the fast-evolving tech landscape with regular updates and insights on Rust, Software Development, and emerging technologies by subscribing to my newsletter on LinkedIn. Subscribe Here
🔗 Connect with Me:
- Medium: Read my articles on Medium and give claps if you find them helpful. It motivates me to keep writing and sharing Rust content. Follow on Medium
- Personal Blog: Discover more on my personal blog, a hub for all my Rust-related content. Visit Blog
- LinkedIn: Join my professional network for more insightful discussions and updates. Connect on LinkedIn
- Twitter: Follow me on Twitter for quick updates and thoughts on Rust programming. Follow on Twitter
Wanna talk? Leave a comment or drop me a message!
All the best,
Luis Soares
luis.soares@linux.com
Senior Software Engineer | Cloud Engineer | SRE | Tech Lead | Rust | Golang | Java | ML AI & Statistics | Web3 & Blockchain